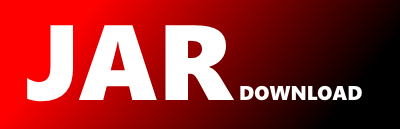
ch.sahits.game.openpatrician.engine.land.city.AutomaticTradingEngine Maven / Gradle / Ivy
package ch.sahits.game.openpatrician.engine.land.city;
import ch.sahits.game.event.data.PeriodicalDailyUpdate;
import ch.sahits.game.openpatrician.clientserverinterface.service.TradeService;
import ch.sahits.game.openpatrician.engine.AbstractEngine;
import ch.sahits.game.openpatrician.engine.event.task.ServerSideTaskFactory;
import ch.sahits.game.openpatrician.model.IBalanceSheet;
import ch.sahits.game.openpatrician.model.building.ETradeType;
import ch.sahits.game.openpatrician.model.building.IAutomatedTrading;
import ch.sahits.game.openpatrician.model.building.ITradingOffice;
import ch.sahits.game.openpatrician.model.building.TradingOfficeList;
import ch.sahits.game.openpatrician.model.city.ICity;
import ch.sahits.game.openpatrician.model.event.BuildingFinished;
import ch.sahits.game.openpatrician.model.event.TimedUpdatableTaskList;
import ch.sahits.game.openpatrician.model.product.EWare;
import ch.sahits.game.openpatrician.utilities.annotation.ClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.EClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.LazySingleton;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.base.Preconditions;
import com.google.common.eventbus.AsyncEventBus;
import com.google.common.eventbus.Subscribe;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
/**
* @author Andi Hotz, (c) Sahits GmbH, 2014
* Created on Dec 23, 2014
*/
@LazySingleton
@ClassCategory(EClassCategory.SINGLETON_BEAN)
public class AutomaticTradingEngine extends AbstractEngine {
@Autowired
@Qualifier("serverClientEventBus")
private AsyncEventBus clientServerEventBus;
@Autowired
private TradingOfficeList offices;
@Autowired
private TimedUpdatableTaskList taskList;
@Autowired
private ServerSideTaskFactory taskFactory;
@Autowired
private TradeService tradeService;
@PreDestroy
private void unregister() {
clientServerEventBus.unregister(this);
}
@PostConstruct
private void initialize() {
clientServerEventBus.register(this);
taskList.add(taskFactory.getDailyAIPlayerCheck());
taskList.add(taskFactory.getPeriodicalDailyUpdateTask());
}
@Override
public List getChildren() {
return new ArrayList<>();
}
/**
* When a traiding office is build add it.
* @param event a new building has been finished
*/
@Subscribe
public void handleTradingOfficeBuilt(BuildingFinished event) {
if (event.getBuilding() instanceof ITradingOffice) {
final ITradingOffice tradingOffice = (ITradingOffice) event.getBuilding();
offices.add(tradingOffice);
Preconditions.checkArgument(!tradingOffice.getSteward().isPresent(), "There must not be a stewart assigned.");
}
}
/**
* Check if the update message is addressed to this instance and find the targeted
* Tradeing office. Execute the buy and sell action on the office.
* @param event daily update
*/
@Subscribe
public void handleDailyUpdates(PeriodicalDailyUpdate event) {
for (ITradingOffice office : offices) {
if (office.getSteward().isPresent()) {
sellAndBuy(office);
}
}
}
/**
* Execute the automatic buying and selling of the automated trading.
* @param office trading office involved in the trading.
*/
@VisibleForTesting
void sellAndBuy(ITradingOffice office) {
Preconditions.checkArgument(office.getSteward().isPresent(), "Stewart must be present");
Preconditions.checkNotNull(office.getOfficeTrading(), "OfficeTrading may not be null");
IAutomatedTrading automatedTrading = office.getOfficeTrading();
IBalanceSheet balanceSheet = office.getCurrentWeek();
// Iterate over all wares and figure out if they should be bought or sold
for (EWare ware : EWare.values()) {
if (automatedTrading.tradingTypeProperty(ware).get() == ETradeType.CITY_OFFICE) {
// buy from the city for at max avgPrice
final ICity city = office.getCity();
int maxAmount = automatedTrading.getAmount(ware);
int maxPrice = automatedTrading.getPrice(ware);
int totalPrice = tradeService.buyFromCityToStorage(office, office.getOwner(), city, ware, maxAmount, Optional.of(maxPrice), office.getSteward());
balanceSheet.updateAutomatedTradingCosts(totalPrice);
}
if (automatedTrading.tradingTypeProperty(ware).get() == ETradeType.OFFICE_CITY) {
final ICity city = office.getCity();
int avgPrice = automatedTrading.getPrice(ware);
int amount = office.getWare(ware).getAmount() - automatedTrading.getAmount(ware);
if (amount > 0) { // check if there is anything to sell
int price = tradeService.sellFromStorageToCity(office, office.getOwner(), city, ware, amount, Optional.of(avgPrice));
balanceSheet.updateAutomatedTradingCosts(price);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy