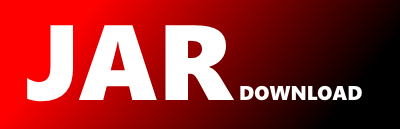
ch.sahits.game.openpatrician.engine.land.city.internal.NextBidTask Maven / Gradle / Ivy
package ch.sahits.game.openpatrician.engine.land.city.internal;
import ch.sahits.game.openpatrician.event.data.AuctionBid;
import ch.sahits.game.openpatrician.event.data.AuctionBidLevel;
import ch.sahits.game.openpatrician.model.IAIPlayer;
import ch.sahits.game.openpatrician.model.city.guild.EBidLevel;
import ch.sahits.game.openpatrician.model.city.guild.IAuction;
import ch.sahits.game.openpatrician.utilities.CancelableRunnable;
import ch.sahits.game.openpatrician.utilities.annotation.ClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.EClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.Prototype;
import com.google.common.eventbus.AsyncEventBus;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Random;
/**
* Task for an auction bid.
* @author Andi Hotz, (c) Sahits GmbH, 2016
* Created on Nov 11, 2016
*/
@Prototype
@ClassCategory({EClassCategory.PROTOTYPE_BEAN,EClassCategory.UNRELEVANT_FOR_DESERIALISATION})
public class NextBidTask implements CancelableRunnable {
@Autowired
@Qualifier("serverClientEventBus")
private AsyncEventBus clientServerEventBus;
@Autowired
private Random rnd;
private final IAuction auction;
public NextBidTask(IAuction auction) {
this.auction = auction;
}
private boolean run = true;
@Override
public void run() {
if (run) {
// Chose if an AI player should place a bid or the level should change
int nextBid = auction.getCurrentBid() + AuctionEngine.BID_DELTA;
EBidLevel bidLevel = auction.getBidLevel();
List biddingPlayers = new ArrayList<>(auction.getBiddingPlayers());
biddingPlayers.remove(auction.getBiddingPlayer());
boolean aiBid = rnd.nextInt(2) % 2 == 0 || bidLevel == EBidLevel.INITIAL;
if (aiBid && !biddingPlayers.isEmpty()) {
Collections.shuffle(biddingPlayers);
IAIPlayer bidder = biddingPlayers.get(0);
clientServerEventBus.post(new AuctionBid(bidder, nextBid, auction));
} else {
EBidLevel nextLevel;
switch (bidLevel) {
case ONE:
nextLevel = EBidLevel.TWO;
break;
case TWO:
nextLevel = EBidLevel.DONE;
break;
default:
throw new IllegalStateException("Levels "+EBidLevel.INITIAL+" and "+EBidLevel.DONE + " are not valid");
}
clientServerEventBus.post(new AuctionBidLevel(bidLevel, nextLevel, auction.getBiddingPlayer(), auction));
}
}
}
@Override
public void cancel() {
run = false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy