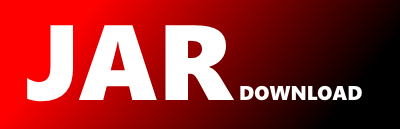
ch.sahits.game.openpatrician.engine.player.HumanPlayerEngine Maven / Gradle / Ivy
package ch.sahits.game.openpatrician.engine.player;
import ch.sahits.game.openpatrician.clientserverinterface.service.ShipService;
import ch.sahits.game.openpatrician.engine.AbstractEngine;
import ch.sahits.game.openpatrician.event.data.BuildingAuctionFinished;
import ch.sahits.game.openpatrician.event.data.BuildingNotAuctioned;
import ch.sahits.game.openpatrician.event.data.ShipAuctionFinished;
import ch.sahits.game.openpatrician.event.data.ShipNotAuctioned;
import ch.sahits.game.openpatrician.model.DisplayMessage;
import ch.sahits.game.openpatrician.model.IHumanPlayer;
import ch.sahits.game.openpatrician.model.IPlayer;
import ch.sahits.game.openpatrician.model.PlayerList;
import ch.sahits.game.openpatrician.model.building.IBuilding;
import ch.sahits.game.openpatrician.model.city.ICity;
import ch.sahits.game.openpatrician.model.event.TargetedEvent;
import ch.sahits.game.openpatrician.model.people.IShipOwner;
import ch.sahits.game.openpatrician.model.ship.IShip;
import ch.sahits.game.openpatrician.utilities.annotation.ClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.EClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.LazySingleton;
import com.google.common.eventbus.AsyncEventBus;
import com.google.common.eventbus.Subscribe;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
/**
* Engine handling automated tasks that are specific to a human player only.
*/
@LazySingleton
@ClassCategory(EClassCategory.SINGLETON_BEAN)
public class HumanPlayerEngine extends AbstractEngine {
@Autowired
private PlayerList players;
@Autowired
@Qualifier("serverClientEventBus")
private AsyncEventBus clientServerEventBus;
@Autowired
private ShipService shipService;
@PostConstruct
private void init() {
clientServerEventBus.register(this);
}
@PreDestroy
private void unregister() {
clientServerEventBus.unregister(this);
}
@Override
public List getChildren() {
return new ArrayList<>();
}
List getHumanPlayers() {
return players.stream().filter(p -> p instanceof IHumanPlayer).collect(Collectors.toList());
}
@Subscribe
public void handleShipAuctionFinished(ShipAuctionFinished event) {
IPlayer owner = event.getNewOwner();
if (owner instanceof IHumanPlayer) {
IShip ship = event.getShip();
ship.setOwner(owner);
owner.addShip(ship);
// Post targeted message
Optional city = shipService.findCity(ship);
DisplayMessage msg;
msg = city.map(iCity ->
new DisplayMessage("ch.sahits.game.openpatrician.engine.player.HumanPlayerEngine.shipAuctioned", ship.getName(), iCity.getName()))
.orElseGet(() -> new DisplayMessage("ch.sahits.game.openpatrician.engine.player.HumanPlayerEngine.shipAuctionedOnSea", ship.getName()));
TargetedEvent targetMsg = new TargetedEvent((IHumanPlayer) owner, msg);
clientServerEventBus.post(targetMsg);
}
}
@Subscribe
public void handleShipNotAuction(ShipNotAuctioned event) {
IShipOwner owner = event.getShip().getOwner();
if (owner instanceof IHumanPlayer) {
// Post targeted message
IShip ship = event.getShip();
((IHumanPlayer) owner).addShip(ship);
Optional city = shipService.findCity(ship);
DisplayMessage msg = new DisplayMessage("ch.sahits.game.openpatrician.engine.player.HumanPlayerEngine.shipNotAuctioned", ship.getName(), city.get().getName());
TargetedEvent targetMsg = new TargetedEvent((IHumanPlayer) event.getNewOwner(), msg);
clientServerEventBus.post(targetMsg);
}
}
@Subscribe
public void handleBuildingAuctionFinished(BuildingAuctionFinished event) {
IPlayer owner = event.getNewOwner();
if (owner instanceof IHumanPlayer) {
// Post targeted message
IBuilding building = event.getBuilding();
building.setOwner(owner);
ICity city = building.getCity();
DisplayMessage msg = new DisplayMessage("ch.sahits.game.openpatrician.engine.player.HumanPlayerEngine.buildingAuctioned", city.getName());
TargetedEvent targetMsg = new TargetedEvent((IHumanPlayer) owner, msg);
clientServerEventBus.post(targetMsg);
}
}
@Subscribe
public void handleBuildingNotAuction(BuildingNotAuctioned event) {
if (event.getNewOwner() instanceof IHumanPlayer) {
// Post targeted message
ICity city = event.getBuilding().getCity();
DisplayMessage msg = new DisplayMessage("ch.sahits.game.openpatrician.engine.player.HumanPlayerEngine.buildingNotAuctioned", city.getName());
TargetedEvent targetMsg = new TargetedEvent((IHumanPlayer) event.getNewOwner(), msg);
clientServerEventBus.post(targetMsg);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy