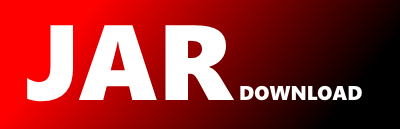
ch.sahits.game.openpatrician.engine.player.strategy.BlockadeStrategy Maven / Gradle / Ivy
package ch.sahits.game.openpatrician.engine.player.strategy;
import ch.sahits.game.openpatrician.clientserverinterface.service.ShipService;
import ch.sahits.game.openpatrician.model.IAIPlayer;
import ch.sahits.game.openpatrician.model.product.ISpecialMission;
import ch.sahits.game.openpatrician.model.ship.INavigableVessel;
import ch.sahits.game.openpatrician.model.ship.IShip;
import ch.sahits.game.openpatrician.utilities.annotation.ClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.EClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.LazySingleton;
import org.springframework.beans.factory.annotation.Autowired;
import java.util.List;
import java.util.Optional;
/**
* The blockade strategy decides which ship to select for sending it to the blockade.
* @author Andi Hotz, (c) Sahits GmbH, 2016
* Created on Jun 23, 2016
*/
@LazySingleton
@ClassCategory(EClassCategory.SINGLETON_BEAN)
public class BlockadeStrategy {
@Autowired
private ShipService shipService;
public Optional selectShip(IAIPlayer player) {
List vessels = player.getSelectableVessels();
IShip strongestShip = null;
int strongest = 0;
for (INavigableVessel vessel : vessels) {
if (vessel instanceof IShip && !(player.getTradeMission(vessel) instanceof ISpecialMission)) {
int strength = shipService.calculateShipsWeaponsStrength(vessel);
if (strongestShip == null) {
strongestShip = (IShip) vessel;
strongest = strength;
} else if (strength > strongest) {
strongestShip = (IShip) vessel;
strongest = strength;
}
}
}
return Optional.ofNullable(strongestShip);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy