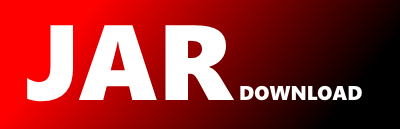
ch.sahits.game.openpatrician.engine.player.strategy.HometownRandomShipConstructionSelectionStrategy Maven / Gradle / Ivy
package ch.sahits.game.openpatrician.engine.player.strategy;
import ch.sahits.game.openpatrician.model.IAIPlayer;
import ch.sahits.game.openpatrician.model.city.ICity;
import ch.sahits.game.openpatrician.model.ship.EShipType;
import ch.sahits.game.openpatrician.model.ship.INavigableVessel;
import ch.sahits.game.openpatrician.utilities.annotation.ClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.EClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.LazySingleton;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
/**
* This strategy builds the biggest possible ship type in the hometown.
* @author Andi Hotz, (c) Sahits GmbH, 2016
* Created on Jul 30, 2016
*/
@LazySingleton
@ClassCategory(EClassCategory.SINGLETON_BEAN)
public class HometownRandomShipConstructionSelectionStrategy extends RandomShipConstructionSelectionStrategy {
@Autowired
private ApplicationContext context;
public HometownRandomShipConstructionSelectionStrategy() {
super(100000, 50, 15000);
}
/**
* {@inheritDoc}
* The build location is always the players hometown.
* @param player
* @return
*/
@Override
public ICity getBuildLocation(IAIPlayer player) {
return player.getHometown();
}
@Override
public void initShipConstruction(IAIPlayer player, INavigableVessel vessel, EShipType shipType) {
CollectConstructionWareStrategy strategy = null;
switch (shipType) {
case HOLK:
strategy = context.getBean(CollectConstructionHometownHolkWareStrategy.class);
break;
case COG:
strategy = context.getBean(CollectConstructionHometownCogWareStrategy.class);
break;
case CRAYER:
strategy = context.getBean(CollectConstructionHometownCrayerWareStrategy.class);
break;
case SNAIKKA:
strategy = context.getBean(CollectConstructionHometownSnaikkaWareStrategy.class);
break;
}
strategy.initializeTradeCycle(player, vessel);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy