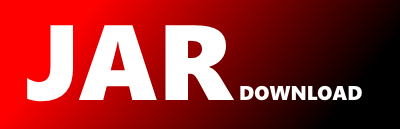
ch.sahits.game.openpatrician.engine.player.tradesteps.CheckForRepairTradeStep Maven / Gradle / Ivy
package ch.sahits.game.openpatrician.engine.player.tradesteps;
import ch.sahits.game.openpatrician.clientserverinterface.service.ShipService;
import ch.sahits.game.openpatrician.model.IAIPlayer;
import ch.sahits.game.openpatrician.model.ICompany;
import ch.sahits.game.openpatrician.model.city.ICity;
import ch.sahits.game.openpatrician.model.player.IAIShipRepairStrategy;
import ch.sahits.game.openpatrician.model.product.ITradeStep;
import ch.sahits.game.openpatrician.model.ship.INavigableVessel;
import ch.sahits.game.openpatrician.model.ship.IShip;
import ch.sahits.game.openpatrician.utilities.annotation.ClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.EClassCategory;
import ch.sahits.game.openpatrician.utilities.annotation.Prototype;
import com.google.common.base.Preconditions;
import com.thoughtworks.xstream.annotations.XStreamOmitField;
import lombok.Setter;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
/**
* Implements the trade step that does the checking if the vessel needs repair and if it does
* sends the ship for repair.
*/
@ClassCategory({EClassCategory.SERIALIZABLE_BEAN, EClassCategory.PROTOTYPE_BEAN})
@Prototype
public class CheckForRepairTradeStep implements ITradeStep {
@XStreamOmitField
private static final Logger LOGGER = LogManager.getLogger(CheckForRepairTradeStep.class);
@Setter
private ICity city;
@Setter
private INavigableVessel vessel;
@Autowired
@XStreamOmitField
private ShipService shipService;
@Override
public boolean execute() {
Preconditions.checkArgument(vessel.getLocation().equals(city.getCoordinates()), "Vessel " +vessel.getName()+" is not in city "+city.getName());
IAIPlayer owner = (IAIPlayer) vessel.getOwner();
IAIShipRepairStrategy repairStrategy = owner.getShipRepairStrategyType().getStrategy();
if (repairStrategy.shouldRepair(vessel, city)) {
repairStrategy.repair(vessel, city);
ICompany company = owner.getCompany();
int costs;
if (vessel instanceof IShip) {
costs = shipService.calculateRepairCosts(city, (IShip) vessel);
} else {
costs = shipService.calculateRepairCostsVessel(city, vessel);
}
company.updateCashDirectly(-costs);
LOGGER.debug("Executed repair check, do NOT proceed for vessel {} in {}", vessel.getName(), city.getName());
return false;
}
LOGGER.debug("Executed repair check, do proceed for vessel {} in {}", vessel.getName(), city.getName());
return true;
}
@Override
public String toString() {
return "CheckForRepairTradeStep in "+city.getName()+" "+((IAIPlayer)vessel.getOwner()).getShipRepairStrategyType();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy