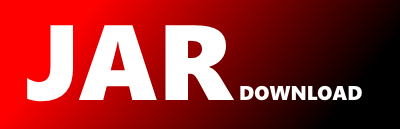
commonMain.ch.softappeal.yass2.serialize.Serializer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yass2-jvm Show documentation
Show all versions of yass2-jvm Show documentation
Yet Another Service Solution
package ch.softappeal.yass2.serialize
import kotlin.math.*
interface Writer {
fun writeByte(byte: Byte)
fun writeBytes(bytes: ByteArray, start: Int = 0, end: Int = bytes.size)
}
interface Reader {
fun readByte(): Byte
fun readBytes(bytes: ByteArray, start: Int = 0, end: Int = bytes.size)
}
interface Serializer {
fun write(writer: Writer, value: Any?)
fun read(reader: Reader): Any?
}
fun Reader.readBytes(length: Int): ByteArray {
var bytes = ByteArray(min(length, 10_000))
var current = 0
while (current < length) { // prevents out-of-memory attack
if (current >= bytes.size) bytes = bytes.copyOf(min(length, 2 * bytes.size))
readBytes(bytes, current, bytes.size)
current = bytes.size
}
return bytes
}
class BytesWriter(val buffer: ByteArray, val start: Int = 0, val end: Int = buffer.size) : Writer {
constructor(size: Int) : this(ByteArray(size))
var current = start
private set
override fun writeByte(byte: Byte) {
require(current < end)
buffer[current++] = byte
}
override fun writeBytes(bytes: ByteArray, start: Int, end: Int) {
require(start <= end)
val newCurrent = current + end - start
require(newCurrent <= this.end)
bytes.copyInto(buffer, current, start, end)
current = newCurrent
}
}
class BytesReader(val buffer: ByteArray, val start: Int = 0, val end: Int = buffer.size) : Reader {
constructor(size: Int) : this(ByteArray(size))
var current = start
private set
override fun readByte(): Byte {
require(current < end)
return buffer[current++]
}
override fun readBytes(bytes: ByteArray, start: Int, end: Int) {
require(start <= end)
val newCurrent = current + end - start
require(newCurrent <= this.end)
buffer.copyInto(bytes, start, current, newCurrent)
current = newCurrent
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy