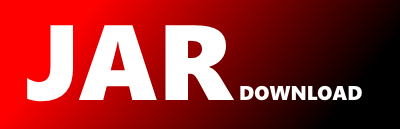
ch.sourcepond.io.checksum.impl.resources.BaseResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of checksum-impl Show documentation
Show all versions of checksum-impl Show documentation
Provides an implementation for the checksum-api bundle.
/*Copyright (C) 2017 Roland Hauser,
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.*/
package ch.sourcepond.io.checksum.impl.resources;
import ch.sourcepond.io.checksum.api.*;
import ch.sourcepond.io.checksum.impl.pools.DigesterPool;
import ch.sourcepond.io.checksum.impl.tasks.TaskFactory;
import ch.sourcepond.io.checksum.impl.tasks.UpdateTask;
import org.slf4j.Logger;
import java.io.IOException;
import java.util.concurrent.Future;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
import static ch.sourcepond.io.checksum.impl.resources.ResourceNotAvailable.RESOURCE_NOT_AVAILABLE;
import static java.util.concurrent.TimeUnit.MILLISECONDS;
import static org.slf4j.LoggerFactory.getLogger;
/**
* Abstract base implementation of the {@link Resource} interface.
*
* @param Type of the accessor necessary to read from the source. This can either be
* {@link ChannelSource} or {@link StreamSource}.
*/
public abstract class BaseResource implements Resource {
private static final Logger LOG = getLogger(BaseResource.class);
final ScheduledExecutorService updateExecutor;
private final A source;
final DigesterPool digesterPool;
final TaskFactory taskFactory;
private Checksum current;
BaseResource(final ScheduledExecutorService pUpdateExecutor,
final A pSource,
final DigesterPool pDigesterPool,
final TaskFactory pTaskFactory) {
updateExecutor = pUpdateExecutor;
source = pSource;
digesterPool = pDigesterPool;
taskFactory = pTaskFactory;
}
public A getSource() {
return source;
}
@Override
public final Algorithm getAlgorithm() {
return digesterPool.getAlgorithm();
}
@Override
public final Future update(final UpdateObserver pObserver) {
return update(0L, pObserver);
}
@Override
public final Future update(final long pIntervalInMilliseconds, final UpdateObserver pObserver) {
return update(MILLISECONDS, pIntervalInMilliseconds, pObserver);
}
@Override
public final Future update(final TimeUnit pUnit, final long pInterval, final UpdateObserver pObserver) {
Future result;
try {
final UpdateTask task = newUpdateTask(pObserver, pUnit, pInterval);
updateExecutor.execute(task);
result = task.getFuture();
} catch (final IOException e) {
LOG.warn(e.getMessage(), e);
result = RESOURCE_NOT_AVAILABLE;
}
return result;
}
abstract UpdateTask newUpdateTask(UpdateObserver pObserver, TimeUnit pUnit, long pInterval) throws IOException;
synchronized Resource initialUpdate() {
setCurrent(new InitialChecksum(update(u -> {})));
return this;
}
public synchronized Checksum getCurrent() {
return current;
}
// Not thread-safe, must be synchronized externally
public void setCurrent(final Checksum pCurrent) {
current = pCurrent;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy