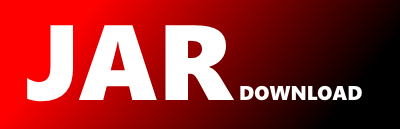
ch.sourcepond.maven.plugin.repobuilder.AdditionalArtifact Maven / Gradle / Ivy
The newest version!
/*Copyright (C) 2015 Roland Hauser,
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.*/
package ch.sourcepond.maven.plugin.repobuilder;
import static com.google.common.io.Files.getFileExtension;
import static org.apache.commons.lang3.StringUtils.isBlank;
import java.io.File;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.artifact.DefaultArtifact;
import org.apache.maven.artifact.handler.DefaultArtifactHandler;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugins.annotations.Parameter;
/**
* Configuration object for additional files to be copied into the dedicated
* Maven-repository.
*
*/
public class AdditionalArtifact {
@Parameter(required = true)
private String artifactId;
@Parameter(required = true)
private String groupId;
@Parameter(required = true)
private String version;
@Parameter
private String classifier;
@Parameter
private String extension;
@Parameter(required = true)
private File file;
/**
* @return
* @throws MojoExecutionException
*/
Artifact toArtifact() throws MojoExecutionException {
final DefaultArtifactHandler handler = new DefaultArtifactHandler();
String extension = this.extension;
if (isBlank(extension)) {
extension = getFileExtension(file.getAbsolutePath());
}
if (isBlank(extension)) {
throw new MojoExecutionException(
"No extension specified; either add an extension to the artifact-file, or, specify parameter 'extension'! Invalid config item: "
+ this);
}
handler.setExtension(extension);
final Artifact artifact = new DefaultArtifact(groupId, artifactId, version, "", handler.getExtension(),
classifier, handler);
artifact.setFile(file);
return artifact;
}
/**
* @param artifactId
*/
void setArtifactId(final String artifactId) {
this.artifactId = artifactId;
}
/**
* @param groupId
*/
void setGroupId(final String groupId) {
this.groupId = groupId;
}
/**
* @param version
*/
void setVersion(final String version) {
this.version = version;
}
/**
* @param classifier
*/
void setClassifier(final String classifier) {
this.classifier = classifier;
}
/**
* @param pExtension
*/
void setExtension(final String pExtension) {
extension = pExtension;
}
/**
* @param pFile
*/
void setFile(final File pFile) {
file = pFile;
}
@Override
public String toString() {
return "[" + groupId + ":" + artifactId + ":" + version + ":" + classifier + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy