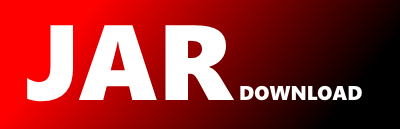
ch.sourcepond.maven.plugin.repobuilder.RepositoryBuilderMojo Maven / Gradle / Ivy
The newest version!
/*Copyright (C) 2015 Roland Hauser,
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.*/
package ch.sourcepond.maven.plugin.repobuilder;
import static org.apache.maven.plugins.annotations.LifecyclePhase.INSTALL;
import static org.apache.maven.plugins.annotations.ResolutionScope.RUNTIME;
import java.io.File;
import java.io.IOException;
import java.nio.file.Path;
import java.util.List;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Component;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
/**
* The only implementation of {@link AbstractMojo} of this plugin. The name of
* the mojo is build-repo and its default-phase is install.
*/
@Mojo(name = "build-repo", defaultPhase = INSTALL, requiresProject = true, requiresDependencyResolution = RUNTIME)
public class RepositoryBuilderMojo extends AbstractMojo {
private static final String DEFAULT_NAME = "${project.build.directory}/dedicated-repository";
static final String REPO_PREFIX = "rpb.";
static final String PROPERTY_RELEASE_REPOSITORY = REPO_PREFIX + "releaseRepository";
static final String PROPERTY_SNAPSHOTS_REPOSITORY = REPO_PREFIX + "snapshotsRepository";
static final String PROPERTY_OUTPUT_DIRECTORY = REPO_PREFIX + "outputDirectory";
static final String PROPERTY_CREATE_ARCHIVE = REPO_PREFIX + "createArchive";
static final String PROPERTY_ARCHIVE_NAME = REPO_PREFIX + "archiveName";
@Component
private RootDirectoryFactory rootDirectoryFactory;
@Component
private RepositorySwitchFactory repositorySwitchFactory;
@Component
private TargetPathFactory targetPathFactory;
@Component
private ArtifactCopier copier;
/**
* Specifies the repository name where to store artifacts.
*/
@Parameter(property = PROPERTY_RELEASE_REPOSITORY)
private String releaseRepository;
/**
* Specifies the repository where to store snapshot artifacts.
*/
@Parameter(property = PROPERTY_SNAPSHOTS_REPOSITORY)
private String snapshotRepository;
/**
*
*/
@Parameter(property = PROPERTY_OUTPUT_DIRECTORY, defaultValue = DEFAULT_NAME, required = true)
private File outputDirectory;
/**
*
*/
@Parameter
private String archiveType;
/**
*
*/
@Parameter
private List additionalArtifacts;
/**
*
*/
@Parameter(defaultValue = "${project}", readonly = true, required = true)
private MavenProject project;
private void copyToTarget(final RepositorySwitch pSwitch, final Artifact pArtifact) throws MojoExecutionException {
final Path repository = pSwitch.getRepository(pArtifact);
final Path copy = targetPathFactory.createTargetPath(repository, pArtifact);
copier.copyToDedicatedRepository(pArtifact.getFile().toPath(), copy);
}
/*
* (non-Javadoc)
*
* @see org.apache.maven.plugin.Mojo#execute()
*/
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
try (final RootDirectory root = rootDirectoryFactory.createRoot(getLog(), outputDirectory, archiveType)) {
final RepositorySwitch repositorySwitch = repositorySwitchFactory.createSwitch(root.getPath(),
snapshotRepository, releaseRepository);
for (final Artifact artifact : project.getArtifacts()) {
copyToTarget(repositorySwitch, artifact);
}
if (additionalArtifacts != null) {
for (final AdditionalArtifact additionalArtifact : additionalArtifacts) {
copyToTarget(repositorySwitch, additionalArtifact.toArtifact());
}
}
getLog().info("Written repository archive to " + root);
} catch (final IOException e) {
throw new MojoExecutionException(e.getMessage(), e);
}
}
/**
* @param pRootDirectoryFactory
*/
void setRootDirectoryFactory(final RootDirectoryFactory pRootDirectoryFactory) {
rootDirectoryFactory = pRootDirectoryFactory;
}
/**
* @param pRepositorySwitchFactory
*/
void setRepositorySwitchFactory(final RepositorySwitchFactory pRepositorySwitchFactory) {
repositorySwitchFactory = pRepositorySwitchFactory;
}
/**
* @param pTargetPathFactory
*/
void setTargetPathFactory(final TargetPathFactory pTargetPathFactory) {
targetPathFactory = pTargetPathFactory;
}
/**
* @param pArtifactCopier
*/
void setArtifactCopier(final ArtifactCopier pArtifactCopier) {
copier = pArtifactCopier;
}
/**
* @param project
*/
void setProject(final MavenProject pProject) {
project = pProject;
}
/**
* @param pReleaseRepository
*/
void setReleaseRepository(final String pReleaseRepository) {
releaseRepository = pReleaseRepository;
}
/**
* @param pSnapshotRepository
*/
void setSnapshotRepository(final String pSnapshotRepository) {
snapshotRepository = pSnapshotRepository;
}
/**
* @param pOutputDirectory
*/
void setOutputDirectory(final File pOutputDirectory) {
outputDirectory = pOutputDirectory;
}
/**
* @param pArchiveType
*/
void setArchiveType(final String pArchiveType) {
archiveType = pArchiveType;
}
/**
* @param pAdditionalArtifacts
*/
void setAddtionalArtifacts(final List pAdditionalArtifacts) {
additionalArtifacts = pAdditionalArtifacts;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy