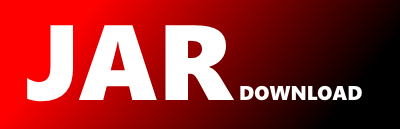
ch.sourcepond.maven.plugin.repobuilder.RootDirectory Maven / Gradle / Ivy
The newest version!
/*Copyright (C) 2015 Roland Hauser,
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.*/
package ch.sourcepond.maven.plugin.repobuilder;
import static net.java.truevfs.access.TVFS.umount;
import java.io.Closeable;
import java.io.IOException;
import java.nio.file.Path;
import org.apache.maven.plugin.logging.Log;
import net.java.truevfs.kernel.spec.FsSyncException;
import net.java.truevfs.kernel.spec.FsSyncWarningException;
/**
* An instance of this class represents the root directory where the Maven
* repositories are stored. Instances of this class must be closed with
* {@link #close()} after they are not needed anymore.
*
*/
class RootDirectory implements Closeable {
private final Log log;
private final Path root;
/**
* Creates a new instance of this class.
*
* @param pLog
* Log instance, must not be {@code null}
* @param pRoot
* Root path, must be a directory and must be {@code null}
*/
public RootDirectory(final Log pLog, final Path pRoot) {
assert pLog != null : "pLog cannot be null";
log = pLog;
root = pRoot;
}
/**
* Returns the root directory held by this object.
*
* @return Path, never {@code null}
*/
public Path getPath() {
return root;
}
/*
* (non-Javadoc)
*
* @see java.io.Closeable#close()
*/
@Override
public void close() throws IOException {
try {
// Releases all resources held by TrueVFS
umount();
} catch (final FsSyncWarningException e) {
log.warn(e.getMessage(), e);
} catch (final FsSyncException e) {
throw new IOException(e.getMessage(), e);
}
}
/*
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return getPath().toAbsolutePath().toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy