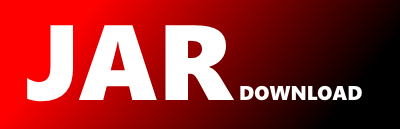
ch.squaredesk.nova.metrics.MetricsDumpToMapConverter Maven / Gradle / Ivy
package ch.squaredesk.nova.metrics;
import ch.squaredesk.nova.tuples.Pair;
import ch.squaredesk.nova.tuples.Tuple3;
import com.codahale.metrics.Metric;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.HashMap;
import java.util.Map;
/**
* This class takes a dump, generated by Nova's Metrics instance and converts them into a map
* that can easily be serialized and deserialized.
*
* The resulting map contains one entry per metric in the dump, each can be looked up by the Metric name. Additionally,
* we add the following
* - timestamp
* - hostName
* - hostAddress
*
* Every Metric itself is again represented as a Map, one MapEntry per attribute. Additionally, we add
* - type
*
*/
public class MetricsDumpToMapConverter {
private static final ObjectMapper objectMapper = new ObjectMapper();
private MetricsDumpToMapConverter() {
}
public static Map convert(MetricsDump dump) {
return convert(dump,null);
}
public static Map convert(MetricsDump dump, Map additionalAttributes) {
HashMap returnValue = new HashMap<>();
dump.metrics.entrySet().stream()
// for each metric, create a tuple containing the type, the name and the metric itself
.map(entry -> new Tuple3<>(
entry.getValue().getClass().getSimpleName(),
entry.getKey(),
entry.getValue()))
// convert each metric to a Map and enrich with our default values, as well as the
// (eventually) passed additionalAttributes
.map(tupleTypeAndNameAndMetric -> {
Map map = toMap(tupleTypeAndNameAndMetric._3);
map.put("type", tupleTypeAndNameAndMetric._1);
if (additionalAttributes != null) {
map.putAll(additionalAttributes);
}
return new Pair<>(tupleTypeAndNameAndMetric._2, map);
})
// and add it to the return value
.forEach(metricNameMapPair -> returnValue.put(metricNameMapPair._1, metricNameMapPair._2));
returnValue.put("timestamp", dump.timestamp);
returnValue.put("hostName", dump.hostName);
returnValue.put("hostAddress", dump.hostAddress);
return returnValue;
}
private static Map toMap(Metric metric) {
if (metric instanceof CompoundMetric) {
return ((CompoundMetric) metric).getValues();
} else {
return objectMapper.convertValue(metric, Map.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy