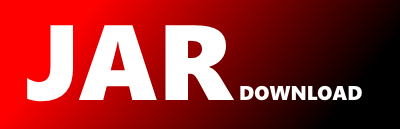
ch.viseon.openOrca.client.ClientOrcaImpl.kt Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2017 viseon gmbh
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ch.viseon.openOrca.client
import ch.viseon.openOrca.share.*
import ch.viseon.openOrca.share.command.CommandApplication
import kodando.rxjs.Rx
import kodando.rxjs.filter
import kodando.rxjs.map
class ClientOrcaImpl(private val commandListExecutor: CommandListExecutor,
private val transmitter: Transmitter) : ClientOrca {
val clientModelStore = ch.viseon.openOrca.share.impl.DefaultPresentationModelStore()
private val eventSubject = kodando.rxjs.Rx.Subject()
private val requestTrackerSubject = kodando.rxjs.Rx.Subject()
override fun executeCommands(commands: Array) {
if (commands.isEmpty()) {
return
}
val appliedCommands = commandListExecutor.execute(clientModelStore, Rx.Observable.from(commands))
val commandsToSend = mutableListOf()
val eventsFromInputCommands = mutableListOf>()
appliedCommands
.subscribe {
if (it.applied) {
eventsFromInputCommands.add(it.events)
}
if (it.sendCommand()) {
commandsToSend.add(it.commandData)
}
}
val responseCommands = transmitter.sendCommands(commandsToSend)
val responseEvents: Rx.IObservable = commandListExecutor.execute(clientModelStore, responseCommands)
val finalObservable = Rx.Observable.merge(
Rx.Observable.from(eventsFromInputCommands.toTypedArray()),
responseEvents
.filter { it.applied }
.map { it.events }
)
requestTrackerSubject.next(RequestEvent.START)
finalObservable
.subscribe({ events: Iterable ->
events.forEach { eventSubject.next(it) }
}, {
if (it is Throwable) {
console.error(ExceptionWithCause("Error during command processing", it))
} else {
console.error("Error during command processing")
console.error(it)
}
}, {
requestTrackerSubject.next(RequestEvent.END)
})
}
override fun observeModelStore(): kodando.rxjs.Rx.IObservable {
return eventSubject
.filter { it is ch.viseon.openOrca.share.ModelStoreChangeEvent }
.map { it as ch.viseon.openOrca.share.ModelStoreChangeEvent }
}
override fun observeModel(modelId: ModelId): kodando.rxjs.Rx.IObservable {
return eventStreamAsPropertyChangeEvent()
.filter { it.modelId == modelId }
}
override fun observeModel(modelType: ModelType): kodando.rxjs.Rx.IObservable {
return eventStreamAsPropertyChangeEvent()
.filter { it.modelType == modelType }
}
override fun observeProperty(modelId: ModelId, propertyName: PropertyName): kodando.rxjs.Rx.IObservable {
return eventStreamAsPropertyChangeEvent()
.filter { it.modelId == modelId }
.filter { it.valueChangeEvent.property == propertyName }
.map { it.valueChangeEvent }
}
private fun eventStreamAsPropertyChangeEvent(): kodando.rxjs.Rx.IObservable {
return eventSubject
.filter { it is ch.viseon.openOrca.share.PropertyChangeEvent }
.map { it as ch.viseon.openOrca.share.PropertyChangeEvent }
}
override fun registerNamedCommand(actionName: String): kodando.rxjs.Rx.IObservable {
return eventSubject
.filter { it is ch.viseon.openOrca.share.ActionEvent }
.map { it as ch.viseon.openOrca.share.ActionEvent }
.filter { it.actionName == actionName }
}
override fun model(modelType: ModelType): Array {
return clientModelStore[modelType].toTypedArray()
}
override fun model(modelId: ModelId): PresentationModel {
return clientModelStore[modelId]
}
override fun contains(modelId: ModelId): Boolean {
return clientModelStore.contains(modelId)
}
override fun contains(modelId: ModelType): Boolean {
return clientModelStore.contains(modelId)
}
override fun getAllModels(): Array {
return clientModelStore.getAllModels().toTypedArray()
}
override fun observeRequest(): Rx.IObservable {
return requestTrackerSubject
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy