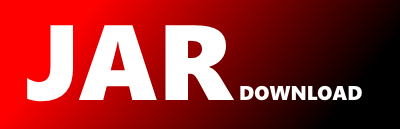
ch.viseon.openOrca.share.Property.kt Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2017 viseon gmbh
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ch.viseon.openOrca.share
/**
* A Property is part of a presentation model. (Actually, a pm only consists of properties)
* A Property can hold a value for a specific `Tag`. E.g. Tag.LABEL => Value for a label,
* Tag.VALUE => Value of a property
*/
class Property(
val name: ch.viseon.openOrca.share.PropertyName,
initialValues: Sequence>,
val clientOnly: Boolean = false,
val clientTags: Set = emptySet()
) {
private val propertyValue2Values: MutableMap = HashMap().apply {
initialValues.forEach { (key, value) -> this[key] = value }
}
/**
* @return the oldValue of the given propertyValue.
*/
operator fun set(tag: Tag, value: Any): Any? {
checkIfValueCanBeSent(tag, value)
val oldValue: Any? = propertyValue2Values[tag]
propertyValue2Values[tag] = value
return oldValue
}
private fun checkIfValueCanBeSent(tag: Tag, value: Any) {
if (!isSupported(value)) {
throw IllegalArgumentException("Not supported value: '$value'. Property: '$name' for Tag '$tag'")
}
}
fun getValuesArray(): Array> {
return propertyValue2Values.entries.asSequence().map { Pair(it.key, it.value) }.toList().toTypedArray()
}
fun getValues(): Sequence> {
return propertyValue2Values.entries.asSequence().map { Pair(it.key, it.value) }
}
operator fun get(tag: Tag = Tag.VALUE): Any {
return propertyValue2Values[tag] ?: throw IllegalArgumentException("No value for tag '$tag'")
}
inline fun getValue(tag: Tag = Tag.VALUE): T {
val get = get(tag)
return get as? T ?: throw IllegalArgumentException("Value is not of type ${T::class}. Actual value '$get' Type: ${get.let { it::class.simpleName }}")
}
override fun toString(): String {
return "$name isClientProperty: $clientOnly values: $propertyValue2Values"
}
fun hasValue(tag: Tag = Tag.VALUE): Boolean {
return propertyValue2Values.containsKey(tag)
}
/**
* Returns `true` if this property is a client property or if the given tag is marked as a client only tag.
*/
fun isClientTag(tag: Tag): Boolean {
return clientOnly || clientTags.contains(tag)
}
override fun equals(other: Any?): Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Property
if (name != other.name) return false
if (clientOnly != other.clientOnly) return false
if (clientTags != other.clientTags) return false
if (propertyValue2Values != other.propertyValue2Values) return false
return true
}
override fun hashCode(): Int {
var result = name.hashCode()
result = 31 * result + clientOnly.hashCode()
result = 31 * result + clientTags.hashCode()
result = 31 * result + propertyValue2Values.hashCode()
return result
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy