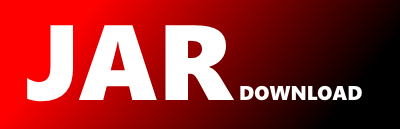
church.i18n.resources.bundles.SingleLocaleMultiResourceBundle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of church.i18n.resources.bundles Show documentation
Show all versions of church.i18n.resources.bundles Show documentation
Project name: church.i18n.resources.bundles
Improved Resource bundles that are able to hold multiple different localization files and provide
functionality to handle multiple language mutations at once.
.
The newest version!
/*
* Copyright (c) 2019 Juraj Jurčo
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and
* associated documentation files (the "Software"), to deal in the Software without restriction,
* including without limitation the rights to use, copy, modify, merge, publish, distribute,
* sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all copies or
* substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT
* NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
* DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package church.i18n.resources.bundles;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Enumeration;
import java.util.List;
import java.util.Locale;
import java.util.Objects;
import java.util.ResourceBundle;
import java.util.stream.Collectors;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Is a resource bundle that is able to handle multiple source files with messages.
*/
public class SingleLocaleMultiResourceBundle extends ResourceBundle implements MultiResourceBundle {
private static final Logger log = LoggerFactory.getLogger(SingleLocaleMultiResourceBundle.class);
private final List bundles = new ArrayList<>();
private final Locale locale;
public SingleLocaleMultiResourceBundle(final Locale locale) {
super();
this.locale = locale;
}
/**
* Gets an object for the given key from this resource bundle. Returns null if this resource
* bundle does not contain an object for the given key. In the case multiple files has contained
* the same key, there is no guarantee of what message will be returned.
*
* @param key The key for the desired object.
* @return The object for the given key, or null.
* @throws NullPointerException If {@code key} is {@code null}.
*/
@Override
protected Object handleGetObject(final String key) {
log.trace("handleGetObject(key = [{}])", key);
return bundles.stream()
.filter(delegate -> delegate.containsKey(key))
.map(delegate -> delegate.getObject(key))
.findFirst().orElse(null);
}
/**
* Returns an enumeration of the keys.
*
* @return An @{code Enumeration} of the keys contained in this @{code ResourceBundle} and its
* parent bundles.
*/
@Override
public Enumeration getKeys() {
log.trace("getKeys()");
return Collections.enumeration(bundles.stream()
.filter(Objects::nonNull)
.flatMap(delegate -> Collections.list(delegate.getKeys()).stream())
.collect(Collectors.toSet()));
}
@Override
public MultiResourceBundle addMessageSources(final String... fileLocations) {
if (fileLocations == null) {
return this;
}
for (final String fileLocation : fileLocations) {
if (fileLocation != null) {
ResourceBundle bundle = ResourceBundle.getBundle(fileLocation, locale);
//this check is needed, because if the locale is not found, ResourceBundle does failover and
//selects some default one. We would like to avoid it so we do not mix locales
if (locale.equals(bundle.getLocale())) {
bundles.add(bundle);
}
}
}
return this;
}
@Override
public Locale getLocale() {
return locale;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy