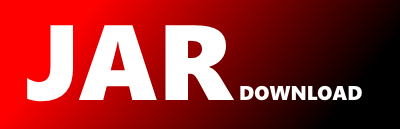
cl.alma.camel.acslog.ACSLogConsumerXML Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-acslog Show documentation
Show all versions of camel-acslog Show documentation
ACS / ALMA Common Software logging Camel Consumer Component
package cl.alma.camel.acslog;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileFilter;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.math.BigInteger;
import org.apache.camel.Exchange;
import org.apache.camel.Message;
import org.apache.camel.Processor;
import org.apache.camel.impl.DefaultConsumer;
import org.apache.camel.impl.DefaultExchange;
import org.apache.camel.impl.DefaultMessage;
import org.apache.commons.io.FilenameUtils;
import com.cosylab.logging.engine.ACS.ACSRemoteErrorListener;
import com.cosylab.logging.engine.ACS.ACSRemoteLogListener;
import com.cosylab.logging.engine.ACS.ACSRemoteRawLogListener;
import com.cosylab.logging.engine.audience.Audience;
import com.cosylab.logging.engine.audience.Audience.AudienceInfo;
import com.cosylab.logging.engine.log.ILogEntry;
import com.cosylab.logging.engine.log.LogField;
import com.cosylab.logging.engine.log.LogTypeHelper;
import alma.acs.logging.engine.io.IOHelper;
import alma.acs.logging.engine.io.IOPorgressListener;
/**
*
* @author atejeda
*
*/
public class ACSLogConsumerXML extends DefaultConsumer implements ACSRemoteLogListener,
ACSRemoteRawLogListener, ACSRemoteErrorListener, IOPorgressListener {
private final ACSLogEndpoint endpoint;
private BigInteger log_preuid = null;
private BigInteger log_parsed = null;
private int files_read = 0;
private String xmlPath = null;
private File xmlDir = null;
private IOHelper ioHelper = null;
/**
* Constructor, set the xml directory path from the endpoint options,
* @param endpoint
* @param processor
*/
public ACSLogConsumerXML(ACSLogEndpoint endpoint, Processor processor) {
super(endpoint, processor);
this.endpoint = endpoint;
this.log_preuid = BigInteger.ZERO;
this.log_parsed= BigInteger.ZERO;
this.xmlPath = this.endpoint.getXml();
}
/**
* Start the common validation files and reading process
* of the log, if is a file, or the logs located at that directory.
*
* Invoke {@link #doStop()} whe all logs files were processed.
*/
@Override
protected void doStart() throws Exception {
log.info("Using directory ", this.xmlPath);
this.xmlDir = new File(this.xmlPath);
if(!this.xmlDir.canRead() || !this.xmlDir.exists()) {
log.info("Can't read " + this.xmlPath + ", exists?");
} else if(this.xmlDir.isFile()) {
this.readLog(this.xmlDir);
} else {
this.readLogs();
}
log.info("*** all xml log files were read ***");
log.info("logs file read :" + this.files_read);
}
/**
* If the endpoint option were a directory, it will start reading
* all logs from that directory with an .xml extension.
*/
public void readLogs() {
try {
for(File nfile : this.xmlDir.listFiles(this.xmlFileFilter())) {
this.readLog(nfile);
}
} catch (Exception e) {
log.error("Can't read the file..." + e.toString());
}
}
/**
* Using ACS (ALMA Common Software) loggin api, it will start reading
* the log xml file.
* @param logFile the xml log to read.
*/
public void readLog(File logFile) {
this.files_read = 0;
this.log_preuid = BigInteger.ZERO;
this.log_parsed = BigInteger.ZERO;
log.info("reading " + logFile.getAbsolutePath());
try {
BufferedReader bufferReader = new BufferedReader(new FileReader(logFile));
this.ioHelper = new IOHelper();
this.ioHelper.setDiscardLevel(this.getLogDiscardLevel());
this.ioHelper.setAudience(this.getAudience());
this.ioHelper.loadLogs(bufferReader, this, this, this, this);
this.files_read++;
} catch (FileNotFoundException e) {
log.error(e.getMessage());
} catch (Exception e) {
log.error(e.getMessage());
}
log.info("logs read : " + this.log_preuid.toString());
log.info("logs parsed : " + this.log_parsed.toString());
}
/**
* It's an anonymous implementation for filtering
* xml files with xml entension.
* @return a file filter for xml file extension.
*/
public FileFilter xmlFileFilter() {
return new FileFilter() {
@Override
public boolean accept(File pathname) {
return pathname.isFile() &&
FilenameUtils.isExtension(pathname.getName(), "xml");
}
};
}
/**
*
* @param logEntry
* @return
*/
public Object wrappedLogEntry(ILogEntry logEntry) {
return new ACSLogEntryWrapper(logEntry, this.endpoint.getOrigin(), this.log_preuid.toString());
}
/**
*
* @param logEntry
* @return
*/
public Exchange createExchange(ILogEntry logEntry) {
Exchange exchange = new DefaultExchange(this.endpoint.getCamelContext(),
this.endpoint.getExchangePattern());
Message message = new DefaultMessage();
if(this.endpoint.getMode().equalsIgnoreCase(ACSLogEndpoint.SEND_MODE_OBJECT)){
logEntry.addData("origin", this.endpoint.getOrigin());
logEntry.addData("uid", ACSLogEntryWrapper.generateUID((Long)logEntry.getField(LogField.TIMESTAMP),
this.log_preuid.toString()));
message.setBody(logEntry);
} else if(this.endpoint.getMode().equalsIgnoreCase(ACSLogEndpoint.SEND_MODE_XMLSTRING)){
logEntry.addData("origin", this.endpoint.getOrigin());
logEntry.addData("uid", ACSLogEntryWrapper.generateUID((Long)logEntry.getField(LogField.TIMESTAMP),
this.log_preuid.toString()));
message.setBody(logEntry.toXMLString());
} else if(this.endpoint.getMode().equalsIgnoreCase(ACSLogEndpoint.SEND_MODE_WRAPPED)){
message.setBody(this.wrappedLogEntry(logEntry));
}
exchange.setIn(message);
return exchange;
}
/**
* Receive a log entry from the xml files, updates the read log count
* and the read log parsed count, also add the "origin" field to the
* data file of the ACS logging api structure, the purpuse is to
* identify the origin of this log, eg.: my-localhost-name.
*
* Also it will create the Camel exchange to be sent to the processor.
*/
@Override
public void logEntryReceived(ILogEntry logEntry) {
this.log_preuid = this.log_preuid.add(BigInteger.ONE);
try {
this.getProcessor().process(this.createExchange(logEntry));
this.log_parsed = this.log_parsed.add(BigInteger.ONE);
} catch (Exception e) {
log.error(e.toString());
e.printStackTrace();
}
}
/**
* Returns the audience for reading the log, defined in the endpoint,
* ACS logging api use this as a filter.
* @return the audience, by default: Enginner.
*/
public Audience getAudience() {
String audienceString = this.endpoint.getAudience();
Audience audience;
audience = AudienceInfo.fromShortName(audienceString).getAudience();
if(audience == null) {
audience = AudienceInfo.ENGINEER.getAudience();
}
return audience;
}
/**
* Returns the discard level for reading the log, defined in the endpoint,
* ACS logging api use this as a filter.
* @return the discard level, by default: Debug.
*/
public LogTypeHelper getLogDiscardLevel() {
String logdiscardString = this.endpoint.getDiscard();
LogTypeHelper logDiscard;
logDiscard = LogTypeHelper.fromLogTypeDescription(logdiscardString);
if(logDiscard == null) {
logDiscard= LogTypeHelper.DEBUG;
}
return logDiscard;
}
/**
* Returns the log level for reading the log, defined in the endpoint,
* ACS logging api use this as a filter.
* @return the log level, by default: Info.
*/
public LogTypeHelper getLogLevel() {
String loglevelString = this.endpoint.getLevel();
LogTypeHelper loglevel;
loglevel = LogTypeHelper.fromLogTypeDescription(loglevelString);
if(loglevel == null) {
loglevel= LogTypeHelper.INFO;
}
return loglevel;
}
/**
* Not implemented and used, forced by the observer interface.
*/
@Override
public void logsRead(int numOfLogs) {}
/**
* Not implemented and used, forced by the observer interface.
*/
@Override
public void xmlEntryReceived(String xmlLogString) {}
/**
* Not implemented and used, forced by the observer interface.
*/
@Override
public void logsWritten(int numOfLogs) {}
/**
* Not implemented and used, forced by the observer interface.
*/
@Override
public void errorReceived(String xml) {}
/**
* Not implemented and used, forced by the observer interface.
*/
@Override
public void bytesRead(long nBytes) {}
/**
* Not implemented and used, forced by the observer interface.
*/
@Override
public void bytesWritten(long nBytes) {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy