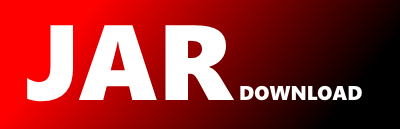
cl.alma.camel.acslog.ACSLogEndpoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-acslog Show documentation
Show all versions of camel-acslog Show documentation
ACS / ALMA Common Software logging Camel Consumer Component
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cl.alma.camel.acslog;
import org.apache.camel.Consumer;
import org.apache.camel.Processor;
import org.apache.camel.Producer;
import org.apache.camel.RuntimeCamelException;
import org.apache.camel.impl.DefaultEndpoint;
import org.apache.camel.spi.UriEndpoint;
import org.apache.camel.spi.UriParam;
/**
* Represents a ACSLog endpoint.
*/
@UriEndpoint(scheme = "acslog")
public class ACSLogEndpoint extends DefaultEndpoint {
public static final String SEND_MODE_WRAPPED = "w";
public static final String SEND_MODE_XMLSTRING = "s";
public static final String SEND_MODE_OBJECT = "o";
@UriParam
private String xml;
@UriParam
private String audience = "Engineer";
@UriParam
private String discard = "Trace";
@UriParam
private String level = "Info";
@UriParam
private String origin = "origin_not_defined";
@UriParam
private String mode = "SEND_MODE_OBJECT";
/**
* Constructor
*/
public ACSLogEndpoint() { }
/**
* @see {@link DefaultEndpoint}
* @param uri
* @param component
*/
public ACSLogEndpoint(String uri, ACSLogComponent component) {
super(uri, component);
}
/**
* This endpoint implementation does not allow a
* producer, just a consumer.
* @see {@link DefaultEndpoint#createProducer()}
*/
public Producer createProducer() throws Exception {
//return new ACSLogProducer(this);
throw new RuntimeCamelException("Cannot produce to a ACSLog endpoint (one way only): " + getEndpointUri());
}
/**
* Creates the consumer, if "xml" was specified in the endpoint
* options, it will create a {@link ACSLogConsumerXML}, otherwise
* it will use a ACSLogConsumerOnline
* @see {@link DefaultEndpoint#createConsumer(Processor)}
*/
public Consumer createConsumer(Processor processor) throws Exception {
ACSLogConsumerXML consumer = new ACSLogConsumerXML(this, processor);
this.configureConsumer(consumer);
return consumer;
}
/**
* @see {@link DefaultEndpoint#isSingleton()}
*/
public boolean isSingleton() {
return true;
}
/**
* @return the xml
*/
public String getXml() {
return xml;
}
/**
* @param xml the xml to set
*/
public void setXml(String xml) {
this.xml = xml;
}
/**
* @return the audience
*/
public String getAudience() {
return audience;
}
/**
* @param audience the audience to set
*/
public void setAudience(String audience) {
this.audience = audience;
}
/**
* @return the discard
*/
public String getDiscard() {
return discard;
}
/**
* @param discard the discard to set
*/
public void setDiscard(String discard) {
this.discard = discard;
}
/**
* @return the level
*/
public String getLevel() {
return level;
}
/**
* @param level the level to set
*/
public void setLevel(String level) {
this.level = level;
}
/**
* @return the location
*/
public String getOrigin() {
return origin.toLowerCase();
}
/**
* @param location the location to set
*/
public void setOrigin(String origin) {
this.origin = origin.toLowerCase();
}
/**
* @return the mode
*/
public String getMode() {
return mode;
}
/**
* @param mode the mode to set
*/
public void setMode(String mode) {
this.mode = mode;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy