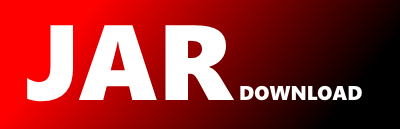
cloud.agileframework.data.common.dao.TableWrapper Maven / Gradle / Ivy
package cloud.agileframework.data.common.dao;
import cloud.agileframework.common.util.object.ObjectUtil;
import java.lang.reflect.AccessibleObject;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import java.util.function.Function;
import java.util.stream.Collectors;
public class TableWrapper {
private final T o;
private final List columns;
private final String tableName;
public TableWrapper(T o, Function, List> toColumnNamesFunction, Function, String> toTableName) {
this.o = o;
Class tClass = (Class) o.getClass();
this.tableName = toTableName.apply(tClass);
columns = toColumnNamesFunction.apply(tClass).stream()
.filter(c -> Arrays.stream(((AccessibleObject) (c.getMember())).getAnnotations()).noneMatch(annotation ->
"Transient".equals(annotation.annotationType().getSimpleName())))
.collect(Collectors.toList());
columns.stream().filter(f -> f.getMember() instanceof Field)
.forEach(f -> f.setValue(Optional.ofNullable(ObjectUtil.getFieldValue(o, (Field) f.getMember()))));
columns.stream().filter(f -> f.getMember() instanceof Method)
.forEach(f -> {
try {
Method method = (Method) f.getMember();
method.setAccessible(true);
f.setValue(Optional.ofNullable(method.invoke(o)));
} catch (IllegalAccessException | InvocationTargetException e) {
e.printStackTrace();
}
f.setValue(Optional.empty());
});
}
public T getO() {
return o;
}
public List getColumns() {
return columns;
}
public String getTableName() {
return tableName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy