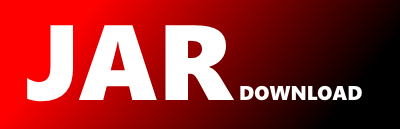
cloud.agileframework.common.util.collection.TreeUtil Maven / Gradle / Ivy
package cloud.agileframework.common.util.collection;
import cloud.agileframework.common.constant.Constant;
import cloud.agileframework.common.util.clazz.ClassUtil;
import cloud.agileframework.common.util.object.ObjectUtil;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import java.io.Serializable;
import java.lang.reflect.Field;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.SortedSet;
import java.util.concurrent.ConcurrentSkipListSet;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* @author 佟盟
* @version 1.0
* @since 1.0
*/
public class TreeUtil {
/**
* 快速构建树形结构
*
* 借助引用,快速构建树形结构,避免了递归的消耗
*
* @param nodes 构建源数据
* @return 树形结构数据集
*/
public static > SortedSet createTree(Collection extends T> nodes, A rootValue, String splitChar, Set fullFieldSet) {
Map, List> children = nodes.stream().filter(node -> {
node.getChildren().clear();
return !Objects.equals(node.getParentId(), rootValue);
}).collect(Collectors.groupingBy(n -> Optional.ofNullable(n.getParentId())));
nodes.forEach(node -> {
final List child = children.get(Optional.ofNullable(node.getId()));
if (child == null) {
return;
}
node.setChildren(new ConcurrentSkipListSet<>(child));
});
//计算full属性值,借助ParentWrapper包裹,通过引用实现快速计算
if (fullFieldSet != null && !fullFieldSet.isEmpty()) {
List> wrapperList = nodes.stream().map(ParentWrapper::new).collect(Collectors.toList());
Map> map = Maps.newConcurrentMap();
wrapperList.forEach(a -> map.put(a.getCurrent().getId(), a));
wrapperList.forEach(a -> {
Object parentId = a.getCurrent().getParentId();
if (parentId == null) {
return;
}
ParentWrapper parentWrapper = map.get(parentId);
if (parentWrapper == null) {
return;
}
a.setParent(parentWrapper);
});
Map