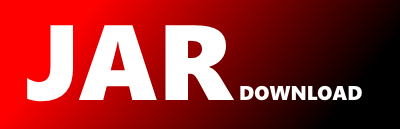
cloud.commandframework.fabric.FabricExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-fabric Show documentation
Show all versions of cloud-fabric Show documentation
Command framework and dispatcher for the JVM
The newest version!
//
// MIT License
//
// Copyright (c) 2022 Alexander Söderberg & Contributors
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
package cloud.commandframework.fabric;
import cloud.commandframework.exceptions.ArgumentParseException;
import cloud.commandframework.exceptions.CommandExecutionException;
import cloud.commandframework.exceptions.InvalidCommandSenderException;
import cloud.commandframework.exceptions.InvalidSyntaxException;
import cloud.commandframework.exceptions.NoPermissionException;
import cloud.commandframework.exceptions.NoSuchCommandException;
import com.mojang.brigadier.Command;
import com.mojang.brigadier.context.CommandContext;
import com.mojang.brigadier.exceptions.CommandSyntaxException;
import com.mojang.logging.LogUtils;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.concurrent.CompletionException;
import java.util.function.BiConsumer;
import java.util.function.Function;
import net.minecraft.class_124;
import net.minecraft.class_2172;
import net.minecraft.class_2558;
import net.minecraft.class_2561;
import net.minecraft.class_2564;
import net.minecraft.class_2568;
import net.minecraft.class_5250;
import org.checkerframework.checker.nullness.qual.NonNull;
import org.slf4j.Logger;
final class FabricExecutor implements Command {
private static final Logger LOGGER = LogUtils.getLogger();
private static final class_2561 NEWLINE = class_2561.method_43470("\n");
private static final String MESSAGE_INTERNAL_ERROR = "An internal error occurred while attempting to perform this command.";
private static final String MESSAGE_NO_PERMS =
"I'm sorry, but you do not have permission to perform this command. "
+ "Please contact the server administrators if you believe that this is in error.";
private static final String MESSAGE_UNKNOWN_COMMAND = "Unknown command. Type \"/help\" for help.";
private final FabricCommandManager manager;
private final Function getName;
private final BiConsumer sendError;
FabricExecutor(
final @NonNull FabricCommandManager manager,
final @NonNull Function getName,
final @NonNull BiConsumer sendError
) {
this.manager = manager;
this.getName = getName;
this.sendError = sendError;
}
@Override
public int run(final @NonNull CommandContext ctx) {
final S source = ctx.getSource();
final String input = ctx.getInput().substring(ctx.getLastChild().getNodes().get(0).getRange().getStart());
final C sender = this.manager.commandSourceMapper().apply(source);
this.manager.executeCommand(sender, input).whenComplete((result, throwable) -> {
if (throwable == null) {
return;
}
if (throwable instanceof CompletionException) {
throwable = throwable.getCause();
}
this.handleThrowable(source, sender, throwable);
});
return com.mojang.brigadier.Command.SINGLE_SUCCESS;
}
private void handleThrowable(final @NonNull S source, final @NonNull C sender, final @NonNull Throwable throwable) {
if (throwable instanceof InvalidSyntaxException) {
this.manager.handleException(
sender,
InvalidSyntaxException.class,
(InvalidSyntaxException) throwable,
(c, e) -> this.sendError.accept(
source,
class_2561.method_43470("Invalid Command Syntax. Correct command syntax is: ")
.method_10852(class_2561.method_43470(String.format("/%s", e.getCorrectSyntax()))
.method_27694(style -> style.method_10977(class_124.field_1080)))
)
);
} else if (throwable instanceof InvalidCommandSenderException) {
this.manager.handleException(
sender,
InvalidCommandSenderException.class,
(InvalidCommandSenderException) throwable,
(c, e) -> this.sendError.accept(source, class_2561.method_43470(throwable.getMessage()))
);
} else if (throwable instanceof NoPermissionException) {
this.manager.handleException(
sender,
NoPermissionException.class,
(NoPermissionException) throwable,
(c, e) -> this.sendError.accept(source, class_2561.method_43470(MESSAGE_NO_PERMS))
);
} else if (throwable instanceof NoSuchCommandException) {
this.manager.handleException(
sender,
NoSuchCommandException.class,
(NoSuchCommandException) throwable,
(c, e) -> this.sendError.accept(source, class_2561.method_43470(MESSAGE_UNKNOWN_COMMAND))
);
} else if (throwable instanceof ArgumentParseException) {
this.manager.handleException(
sender,
ArgumentParseException.class,
(ArgumentParseException) throwable,
(c, e) -> {
if (throwable.getCause() instanceof CommandSyntaxException) {
this.sendError.accept(source, class_2561.method_43470("Invalid Command Argument: ")
.method_10852(class_2561.method_43470("")
.method_10852(class_2564
.method_10883(((CommandSyntaxException) throwable.getCause()).getRawMessage()))
.method_27692(class_124.field_1080)));
} else {
this.sendError.accept(source, class_2561.method_43470("Invalid Command Argument: ")
.method_10852(class_2561.method_43470(throwable.getCause().getMessage())
.method_27692(class_124.field_1080)));
}
}
);
} else if (throwable instanceof CommandExecutionException) {
this.manager.handleException(
sender,
CommandExecutionException.class,
(CommandExecutionException) throwable,
(c, e) -> {
this.sendError.accept(source, this.decorateHoverStacktrace(
class_2561.method_43470(MESSAGE_INTERNAL_ERROR),
throwable.getCause(),
sender
));
LOGGER.warn(
"Error occurred while executing command for user {}:",
this.getName.apply(source),
throwable.getCause()
);
}
);
} else {
this.sendError.accept(source, this.decorateHoverStacktrace(
class_2561.method_43470(MESSAGE_INTERNAL_ERROR),
throwable,
sender
));
LOGGER.warn("Error occurred while executing command for user {}:", this.getName.apply(source), throwable);
}
}
private class_5250 decorateHoverStacktrace(final class_5250 input, final Throwable cause, final C sender) {
if (!this.manager.hasPermission(sender, "cloud.hover-stacktrace")) {
return input;
}
final StringWriter writer = new StringWriter();
cause.printStackTrace(new PrintWriter(writer));
final String stackTrace = writer.toString().replace("\t", " ");
return input.method_27694(style -> style
.method_10949(new class_2568(
class_2568.class_5247.field_24342,
class_2561.method_43470(stackTrace)
.method_10852(NEWLINE)
.method_10852(class_2561.method_43470(" Click to copy")
.method_27694(s2 -> s2.method_10977(class_124.field_1080).method_10978(true)))
))
.method_10958(new class_2558(
class_2558.class_2559.field_21462,
stackTrace
)));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy