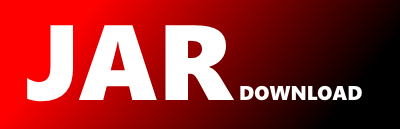
cloud.commandframework.fabric.argument.ItemInputArgument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-fabric Show documentation
Show all versions of cloud-fabric Show documentation
Command framework and dispatcher for the JVM
The newest version!
//
// MIT License
//
// Copyright (c) 2022 Alexander Söderberg & Contributors
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
package cloud.commandframework.fabric.argument;
import cloud.commandframework.ArgumentDescription;
import cloud.commandframework.arguments.CommandArgument;
import cloud.commandframework.context.CommandContext;
import java.util.List;
import java.util.function.BiFunction;
import net.minecraft.class_1799;
import net.minecraft.class_2287;
import net.minecraft.class_2290;
import net.minecraft.class_7923;
import org.checkerframework.checker.nullness.qual.NonNull;
import org.checkerframework.checker.nullness.qual.Nullable;
/**
* An argument parsing an item identifier and optional extra NBT data into an {@link class_2290}.
*
* @param the sender type
* @since 1.5.0
*/
public final class ItemInputArgument extends CommandArgument {
ItemInputArgument(
final boolean required,
final @NonNull String name,
final @NonNull String defaultValue,
final @Nullable BiFunction, String, List> suggestionsProvider,
final @NonNull ArgumentDescription defaultDescription
) {
super(
required,
name,
FabricArgumentParsers.contextual(class_2287::method_9776),
defaultValue,
class_2290.class,
suggestionsProvider,
defaultDescription
);
}
/**
* Create a new {@link cloud.commandframework.fabric.argument.ItemInputArgument.Builder}.
*
* @param name Name of the argument
* @param Command sender type
* @return Created builder
* @since 1.5.0
*/
public static @NonNull cloud.commandframework.fabric.argument.ItemInputArgument.Builder builder(final @NonNull String name) {
return new cloud.commandframework.fabric.argument.ItemInputArgument.Builder<>(name);
}
/**
* Create a new required {@link ItemInputArgument}.
*
* @param name Component name
* @param Command sender type
* @return Created argument
* @since 1.5.0
*/
public static @NonNull ItemInputArgument of(final @NonNull String name) {
return ItemInputArgument.builder(name).asRequired().build();
}
/**
* Create a new optional {@link ItemInputArgument}.
*
* @param name Component name
* @param Command sender type
* @return Created argument
* @since 1.5.0
*/
public static @NonNull ItemInputArgument optional(final @NonNull String name) {
return ItemInputArgument.builder(name).asOptional().build();
}
/**
* Create a new optional {@link ItemInputArgument} with the specified default value.
*
* @param name Argument name
* @param defaultValue Default value
* @param Command sender type
* @return Created argument
* @since 1.5.0
*/
public static @NonNull ItemInputArgument optional(final @NonNull String name, final @NonNull class_1799 defaultValue) {
return ItemInputArgument.builder(name).asOptionalWithDefault(defaultValue).build();
}
/**
* Builder for {@link ItemInputArgument}.
*
* @param sender type
* @since 1.5.0
*/
public static final class Builder extends TypedBuilder> {
Builder(final @NonNull String name) {
super(class_2290.class, name);
}
/**
* Build a new {@link ItemInputArgument}.
*
* @return Constructed argument
* @since 1.5.0
*/
@Override
public @NonNull ItemInputArgument build() {
return new ItemInputArgument<>(
this.isRequired(),
this.getName(),
this.getDefaultValue(),
this.getSuggestionsProvider(),
this.getDefaultDescription()
);
}
/**
* Sets the command argument to be optional, with the specified default value.
*
* @param defaultValue default value
* @return this builder
* @see CommandArgument.Builder#asOptionalWithDefault(String)
* @since 1.5.0
*/
public @NonNull cloud.commandframework.fabric.argument.ItemInputArgument.Builder asOptionalWithDefault(final @NonNull class_1799 defaultValue) {
final String serializedDefault;
if (defaultValue.method_7985()) {
serializedDefault = class_7923.field_41178.method_10221(defaultValue.method_7909()) + defaultValue.method_7969().toString();
} else {
serializedDefault = class_7923.field_41178.method_10221(defaultValue.method_7909()).toString();
}
return this.asOptionalWithDefault(serializedDefault);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy