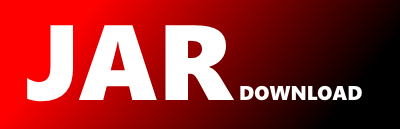
cloud.commandframework.fabric.argument.RegistryEntryArgument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-fabric Show documentation
Show all versions of cloud-fabric Show documentation
Command framework and dispatcher for the JVM
The newest version!
//
// MIT License
//
// Copyright (c) 2022 Alexander Söderberg & Contributors
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
package cloud.commandframework.fabric.argument;
import cloud.commandframework.ArgumentDescription;
import cloud.commandframework.arguments.CommandArgument;
import cloud.commandframework.arguments.parser.ArgumentParseResult;
import cloud.commandframework.arguments.parser.ArgumentParser;
import cloud.commandframework.captions.CaptionVariable;
import cloud.commandframework.context.CommandContext;
import cloud.commandframework.exceptions.parsing.NoInputProvidedException;
import cloud.commandframework.exceptions.parsing.ParserException;
import cloud.commandframework.fabric.FabricCaptionKeys;
import cloud.commandframework.fabric.FabricCommandContextKeys;
import com.mojang.brigadier.StringReader;
import com.mojang.brigadier.exceptions.CommandSyntaxException;
import io.leangen.geantyref.TypeToken;
import java.util.ArrayList;
import java.util.List;
import java.util.Queue;
import java.util.Set;
import java.util.function.BiFunction;
import net.minecraft.class_2172;
import net.minecraft.class_2378;
import net.minecraft.class_2960;
import net.minecraft.class_5321;
import org.checkerframework.checker.nullness.qual.NonNull;
import org.checkerframework.checker.nullness.qual.Nullable;
import static java.util.Objects.requireNonNull;
/**
* Argument for getting a values from a {@link class_2378}.
*
* Both static and dynamic registries are supported.
*
* @param the command sender type
* @param the registry entry type
* @since 1.5.0
*/
public class RegistryEntryArgument extends CommandArgument {
private static final String NAMESPACE_MINECRAFT = "minecraft";
RegistryEntryArgument(
final boolean required,
final @NonNull String name,
final @NonNull class_5321 extends class_2378> registry,
final @NonNull String defaultValue,
final @NonNull TypeToken valueType,
final @Nullable BiFunction, String, List> suggestionsProvider,
final @NonNull ArgumentDescription defaultDescription
) {
super(
required,
name,
new Parser<>(registry),
defaultValue,
valueType,
suggestionsProvider,
defaultDescription
);
}
/**
* Create a new {@link cloud.commandframework.fabric.argument.RegistryEntryArgument.Builder}.
*
* @param name Name of the argument
* @param type The type of registry entry
* @param registry A key for the registry to get values from
* @param Command sender type
* @param Registry entry type
* @return Created builder
* @since 1.5.0
*/
public static RegistryEntryArgument.@NonNull Builder newBuilder(
final @NonNull String name,
final @NonNull Class type,
final @NonNull class_5321 extends class_2378> registry
) {
return new cloud.commandframework.fabric.argument.RegistryEntryArgument.Builder<>(registry, type, name);
}
/**
* Create a new {@link cloud.commandframework.fabric.argument.RegistryEntryArgument.Builder}.
*
* @param name Name of the argument
* @param type The type of registry entry
* @param registry A key for the registry to get values from
* @param Command sender type
* @param Registry entry type
* @return Created builder
* @since 1.5.0
*/
public static RegistryEntryArgument.@NonNull Builder newBuilder(
final @NonNull String name,
final @NonNull TypeToken type,
final @NonNull class_5321 extends class_2378> registry
) {
return new cloud.commandframework.fabric.argument.RegistryEntryArgument.Builder<>(registry, type, name);
}
/**
* Create a new required {@link RegistryEntryArgument}.
*
* @param name Argument name
* @param type The type of registry entry
* @param registry A key for the registry to get values from
* @param Command sender type
* @param Registry entry type
* @return Created argument
* @since 1.5.0
*/
public static @NonNull RegistryEntryArgument of(
final @NonNull String name,
final @NonNull Class type,
final @NonNull class_5321 extends class_2378> registry
) {
return RegistryEntryArgument.newBuilder(name, type, registry).asRequired().build();
}
/**
* Create a new optional {@link RegistryEntryArgument}.
*
* @param name Argument name
* @param type The type of registry entry
* @param registry A key for the registry to get values from
* @param Command sender type
* @param Registry entry type
* @return Created argument
* @since 1.5.0
*/
public static @NonNull RegistryEntryArgument optional(
final @NonNull String name,
final @NonNull Class type,
final @NonNull class_5321 extends class_2378> registry
) {
return RegistryEntryArgument.newBuilder(name, type, registry).asOptional().build();
}
/**
* Create a new optional {@link RegistryEntryArgument} with the specified default value.
*
* @param name Argument name
* @param type The type of registry entry
* @param registry A key for the registry to get values from
* @param defaultValue Default value
* @param Command sender type
* @param Registry entry type
* @return Created argument
* @since 1.5.0
*/
public static @NonNull RegistryEntryArgument optional(
final @NonNull String name,
final @NonNull Class type,
final @NonNull class_5321 extends class_2378> registry,
final @NonNull class_5321 defaultValue
) {
return RegistryEntryArgument.newBuilder(name, type, registry)
.asOptionalWithDefault(defaultValue)
.build();
}
/**
* A parser for values stored in a {@link class_2378}.
*
* @param Command sender type
* @param Registry entry type
* @since 1.5.0
*/
public static final class Parser implements ArgumentParser {
private final class_5321 extends class_2378> registryIdent;
/**
* Create a new {@link Parser}.
*
* @param registryIdent the registry identifier
* @since 1.5.0
*/
public Parser(final class_5321 extends class_2378> registryIdent) {
this.registryIdent = requireNonNull(registryIdent, "registryIdent");
}
@Override
public @NonNull ArgumentParseResult<@NonNull V> parse(
@NonNull final CommandContext<@NonNull C> commandContext,
@NonNull final Queue<@NonNull String> inputQueue
) {
final String possibleIdentifier = inputQueue.peek();
if (possibleIdentifier == null) {
return ArgumentParseResult.failure(new NoInputProvidedException(RegistryEntryArgument.class, commandContext));
}
final class_2960 key;
try {
key = class_2960.method_12835(new StringReader(possibleIdentifier));
} catch (final CommandSyntaxException ex) {
return ArgumentParseResult.failure(ex);
}
inputQueue.poll();
final class_2378 registry = this.resolveRegistry(commandContext);
if (registry == null) {
return ArgumentParseResult.failure(new IllegalArgumentException("Unknown registry " + this.registryIdent));
}
final V entry = registry.method_10223(key);
if (entry == null) {
return ArgumentParseResult.failure(new UnknownEntryException(commandContext, key, this.registryIdent));
}
return ArgumentParseResult.success(entry);
}
class_2378 resolveRegistry(final CommandContext ctx) {
final class_2172 reverseMapped = ctx.get(FabricCommandContextKeys.NATIVE_COMMAND_SOURCE);
return reverseMapped.method_30497().method_33310(this.registryIdent).orElse(null);
}
@Override
public @NonNull List<@NonNull String> suggestions(
final @NonNull CommandContext commandContext,
final @NonNull String input
) {
final Set ids = this.resolveRegistry(commandContext).method_10235();
final List results = new ArrayList<>(ids.size());
for (final class_2960 entry : ids) {
if (entry.method_12836().equals(NAMESPACE_MINECRAFT)) {
results.add(entry.method_12832());
}
results.add(entry.toString());
}
return results;
}
@Override
public boolean isContextFree() {
return true;
}
/**
* Get the registry associated with this parser.
*
* @return the registry
* @since 1.5.0
*/
public class_5321 extends class_2378>> registryKey() {
return this.registryIdent;
}
}
/**
* A builder for {@link RegistryEntryArgument}.
*
* @param The sender type
* @param The registry value type
* @since 1.5.0
*/
public static final class Builder extends CommandArgument.TypedBuilder> {
private final class_5321 extends class_2378> registryIdent;
Builder(
final class_5321 extends class_2378> key,
final @NonNull Class valueType,
final @NonNull String name
) {
super(valueType, name);
this.registryIdent = key;
}
Builder(
final class_5321 extends class_2378> key,
final @NonNull TypeToken valueType,
final @NonNull String name
) {
super(valueType, name);
this.registryIdent = key;
}
@Override
public @NonNull RegistryEntryArgument<@NonNull C, @NonNull V> build() {
return new RegistryEntryArgument<>(
this.isRequired(),
this.getName(),
this.registryIdent,
this.getDefaultValue(),
this.getValueType(),
this.getSuggestionsProvider(),
this.getDefaultDescription()
);
}
/**
* Sets the command argument to be optional, with the specified default value.
*
* @param defaultValue default value
* @return this builder
* @see CommandArgument.Builder#asOptionalWithDefault(String)
* @since 1.5.0
*/
public @NonNull cloud.commandframework.fabric.argument.RegistryEntryArgument.Builder asOptionalWithDefault(final @NonNull class_5321 defaultValue) {
return this.asOptionalWithDefault(defaultValue.method_29177().toString());
}
}
/**
* An exception thrown when an entry in a registry could not be found.
*
* @since 1.5.0
*/
private static final class UnknownEntryException extends ParserException {
private static final long serialVersionUID = 7694424294461849903L;
UnknownEntryException(
final CommandContext> context,
final class_2960 key,
final class_5321 extends class_2378>> registry
) {
super(
RegistryEntryArgument.class,
context,
FabricCaptionKeys.ARGUMENT_PARSE_FAILURE_REGISTRY_ENTRY_UNKNOWN_ENTRY,
CaptionVariable.of("id", key.toString()),
CaptionVariable.of("registry", registry.toString())
);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy