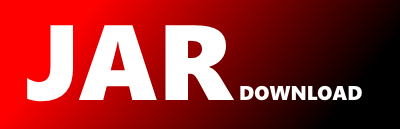
cloud.commandframework.jda.parsers.ChannelArgument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-jda Show documentation
Show all versions of cloud-jda Show documentation
Command framework and dispatcher for the JVM
//
// MIT License
//
// Copyright (c) 2020 Alexander Söderberg & Contributors
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
package cloud.commandframework.jda.parsers;
import cloud.commandframework.arguments.CommandArgument;
import cloud.commandframework.arguments.parser.ArgumentParseResult;
import cloud.commandframework.arguments.parser.ArgumentParser;
import cloud.commandframework.context.CommandContext;
import net.dv8tion.jda.api.entities.MessageChannel;
import net.dv8tion.jda.api.entities.TextChannel;
import net.dv8tion.jda.api.events.message.MessageReceivedEvent;
import org.checkerframework.checker.nullness.qual.NonNull;
import org.jetbrains.annotations.NotNull;
import java.util.ArrayList;
import java.util.List;
import java.util.Queue;
/**
* Command Argument for {@link MessageChannel}
*
* @param Command sender type
*/
@SuppressWarnings("unused")
public final class ChannelArgument extends CommandArgument {
private final List modes;
private ChannelArgument(
final boolean required, final @NonNull String name,
final @NonNull List modes
) {
super(required, name, new MessageParser<>(modes), MessageChannel.class);
this.modes = modes;
}
/**
* Create a new builder
*
* @param name Name of the component
* @param Command sender type
* @return Created builder
*/
public static @NonNull Builder newBuilder(final @NonNull String name) {
return new Builder<>(name);
}
/**
* Create a new required command component
*
* @param name Component name
* @param Command sender type
* @return Created component
*/
public static @NonNull CommandArgument of(final @NonNull String name) {
return ChannelArgument.newBuilder(name).asRequired().build();
}
/**
* Create a new optional command component
*
* @param name Component name
* @param Command sender type
* @return Created component
*/
public static @NonNull CommandArgument optional(final @NonNull String name) {
return ChannelArgument.newBuilder(name).asOptional().build();
}
/**
* Get the modes enabled on the parser
*
* @return List of Modes
*/
public @NotNull List getModes() {
return modes;
}
public enum ParserMode {
MENTION,
ID,
NAME
}
public static final class Builder extends CommandArgument.Builder {
private List modes = new ArrayList<>();
protected Builder(final @NonNull String name) {
super(MessageChannel.class, name);
}
/**
* Set the modes for the parsers to use
*
* @param modes List of Modes
* @return Builder instance
*/
public @NonNull Builder withParsers(final @NonNull List modes) {
this.modes = modes;
return this;
}
/**
* Builder a new example component
*
* @return Constructed component
*/
@Override
public @NonNull ChannelArgument build() {
return new ChannelArgument<>(this.isRequired(), this.getName(), modes);
}
}
public static final class MessageParser implements ArgumentParser {
private final List modes;
private MessageParser(final @NonNull List modes) {
this.modes = modes;
}
@Override
public @NonNull ArgumentParseResult parse(
final @NonNull CommandContext commandContext,
final @NonNull Queue<@NonNull String> inputQueue
) {
final String input = inputQueue.peek();
if (input == null) {
return ArgumentParseResult.failure(new NullPointerException("No input was provided"));
}
final MessageReceivedEvent event = commandContext.get("MessageReceivedEvent");
Exception exception = null;
if (modes.contains(ParserMode.MENTION)) {
if (input.startsWith("<#") || modes.size() == 1) {
final String id = input.substring(2, input.length() - 1);
try {
final ArgumentParseResult channel = this.channelFromId(event, input, id);
inputQueue.remove();
return channel;
} catch (final ChannelNotFoundException | NumberFormatException e) {
exception = e;
}
}
}
if (modes.contains(ParserMode.ID)) {
try {
final ArgumentParseResult result = this.channelFromId(event, input, input);
inputQueue.remove();
return result;
} catch (final ChannelNotFoundException | NumberFormatException e) {
exception = e;
}
}
if (modes.contains(ParserMode.NAME)) {
final List channels = event.getGuild().getTextChannelsByName(input, true);
if (channels.size() == 0) {
exception = new ChannelNotFoundException(input);
} else if (channels.size() > 1) {
exception = new TooManyChannelsFoundParseException(input);
} else {
inputQueue.remove();
return ArgumentParseResult.success(channels.get(0));
}
}
assert exception != null;
return ArgumentParseResult.failure(exception);
}
@Override
public boolean isContextFree() {
return true;
}
private @NonNull ArgumentParseResult channelFromId(
final @NonNull MessageReceivedEvent event,
final @NonNull String input,
final @NonNull String id
)
throws ChannelNotFoundException, NumberFormatException {
final MessageChannel channel = event.getGuild().getTextChannelById(id);
if (channel == null) {
throw new ChannelNotFoundException(input);
}
return ArgumentParseResult.success(channel);
}
}
public static class ChannelParseException extends IllegalArgumentException {
private final String input;
/**
* Construct a new channel parse exception
*
* @param input String input
*/
public ChannelParseException(final @NonNull String input) {
this.input = input;
}
/**
* Get the users input
*
* @return users input
*/
public final @NonNull String getInput() {
return input;
}
}
public static final class TooManyChannelsFoundParseException extends ChannelParseException {
/**
* Construct a new channel parse exception
*
* @param input String input
*/
public TooManyChannelsFoundParseException(final @NonNull String input) {
super(input);
}
@Override
public @NonNull String getMessage() {
return String.format("Too many channels found for '%s'.", getInput());
}
}
public static final class ChannelNotFoundException extends ChannelParseException {
/**
* Construct a new channel parse exception
*
* @param input String input
*/
public ChannelNotFoundException(final @NonNull String input) {
super(input);
}
@Override
public @NonNull String getMessage() {
return String.format("Channel not found for '%s'.", getInput());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy