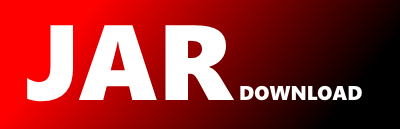
cloud.localstack.LambdaExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of localstack-utils Show documentation
Show all versions of localstack-utils Show documentation
Java utilities for the LocalStack platform.
package cloud.localstack;
import cloud.localstack.lambda.DDBEventParser;
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
import com.amazonaws.services.lambda.runtime.RequestStreamHandler;
import com.amazonaws.services.lambda.runtime.events.KinesisEvent;
import com.amazonaws.services.lambda.runtime.events.KinesisEvent.KinesisEventRecord;
import com.amazonaws.services.lambda.runtime.events.KinesisEvent.Record;
import com.amazonaws.services.lambda.runtime.events.SNSEvent;
import com.amazonaws.util.StringInputStream;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.apache.commons.lang3.StringUtils;
import org.joda.time.DateTime;
import java.io.ByteArrayOutputStream;
import java.io.OutputStream;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.nio.ByteBuffer;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.*;
import java.util.stream.Collectors;
/**
* Simple implementation of a Java Lambda function executor.
*
* @author Waldemar Hummer
*/
@SuppressWarnings("restriction")
public class LambdaExecutor {
@SuppressWarnings("unchecked")
public static void main(String[] args) throws Exception {
if(args.length < 2) {
System.err.println("Usage: java " + LambdaExecutor.class.getSimpleName() +
" ");
System.exit(1);
}
String fileContent = readFile(args[1]);
ObjectMapper reader = new ObjectMapper();
Map map = reader.readerFor(Map.class).readValue(fileContent);
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy