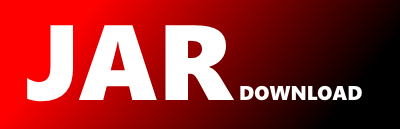
cloud.prefab.client.config.ConfigChangeEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of prefab-cloud-java Show documentation
Show all versions of prefab-cloud-java Show documentation
API Client for https://prefab.cloud: rate limits, feature flags and semaphores as a service
The newest version!
package cloud.prefab.client.config;
import cloud.prefab.domain.Prefab;
import cloud.prefab.domain.Prefab.ConfigValue;
import java.util.Objects;
import java.util.Optional;
import java.util.StringJoiner;
public class ConfigChangeEvent {
private final String key;
private final Optional oldValue;
private final Optional newValue;
public ConfigChangeEvent(
String key,
Optional oldValue,
Optional newValue
) {
this.key = key;
this.oldValue = oldValue;
this.newValue = newValue;
}
public String getKey() {
return key;
}
public Optional getOldValue() {
return oldValue;
}
public Optional getNewValue() {
return newValue;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj instanceof ConfigChangeEvent) {
final ConfigChangeEvent that = (ConfigChangeEvent) obj;
return (
Objects.equals(this.key, that.key) &&
Objects.equals(this.oldValue, that.oldValue) &&
Objects.equals(this.newValue, that.newValue)
);
}
return false;
}
@Override
public int hashCode() {
return Objects.hash(key, oldValue, newValue);
}
@Override
public String toString() {
return new StringJoiner(", ", "ConfigChangeEvent[", "]")
.add("key='" + key + "'")
.add("oldValue=" + oldValue)
.add("newValue=" + newValue)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy