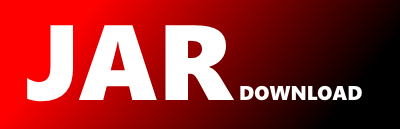
cloud.tianai.rpc.springboot.processor.AnnotationBeanProcessor Maven / Gradle / Ivy
package cloud.tianai.rpc.springboot.processor;
import cloud.tianai.remoting.api.RpcInvocationPostProcessor;
import cloud.tianai.rpc.common.exception.RpcException;
import cloud.tianai.rpc.common.util.CollectionUtils;
import cloud.tianai.rpc.core.bootstrap.ServerBootstrap;
import cloud.tianai.rpc.core.client.proxy.RpcProxy;
import cloud.tianai.rpc.core.client.proxy.RpcProxyFactory;
import cloud.tianai.rpc.core.client.proxy.RpcProxyType;
import cloud.tianai.rpc.core.configuration.RpcClientConfiguration;
import cloud.tianai.rpc.core.configuration.RpcServerConfiguration;
import cloud.tianai.rpc.core.template.RpcClientPostProcessor;
import cloud.tianai.rpc.springboot.annotation.RpcConsumer;
import cloud.tianai.rpc.springboot.annotation.RpcProvider;
import cloud.tianai.rpc.springboot.properties.RpcConsumerProperties;
import cloud.tianai.rpc.springboot.properties.RpcProperties;
import cloud.tianai.rpc.springboot.properties.RpcProviderProperties;
import cloud.tianai.rpc.springboot.properties.RpcReqistryProperties;
import lombok.extern.slf4j.Slf4j;
import org.springframework.aop.support.AopUtils;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.BeanFactory;
import org.springframework.beans.factory.BeanFactoryAware;
import org.springframework.beans.factory.NoSuchBeanDefinitionException;
import org.springframework.beans.factory.config.BeanPostProcessor;
import org.springframework.beans.factory.config.ConfigurableListableBeanFactory;
import org.springframework.boot.context.event.ApplicationStartedEvent;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.context.ApplicationListener;
import org.springframework.context.support.AbstractApplicationContext;
import org.springframework.core.annotation.AnnotationUtils;
import java.lang.reflect.Field;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* @Author: 天爱有情
* @Date: 2020/02/08 15:18
* @Description: @RpcProvider 和 @RpcConsumer 的解析器
*/
@Slf4j
public class AnnotationBeanProcessor implements BeanPostProcessor, ApplicationContextAware, BeanFactoryAware, ApplicationListener {
private RpcClientConfiguration prop;
private RpcProperties rpcProperties;
private RpcConsumerProperties rpcConsumerProperties;
private RpcReqistryProperties rpcReqistryProperties;
private RpcProviderProperties rpcProviderProperties;
private AbstractApplicationContext applicationContext;
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy