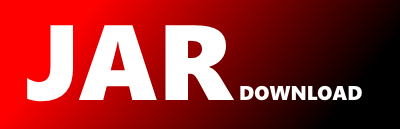
club.chlab.mybatis.provider.SelectEntityProvider Maven / Gradle / Ivy
The newest version!
package club.chlab.mybatis.provider;
import java.lang.reflect.Field;
import org.apache.ibatis.jdbc.SQL;
import club.chlab.mybatis.annotations.Column;
/**
* mybatis select entity provider
* @author jch
*
*/
public class SelectEntityProvider extends BaseProvider {
public String select(Object entity,Field...orderby) {
Class> cls = entity.getClass();
SQL sql = new SQL();
sql.FROM(getTableName(entity));
Field[] fields = cls.getDeclaredFields();
for (int i = 0; i < fields.length; i++) {
Field field = fields[i];
if (field.isAnnotationPresent(Column.class)
&& !isEmpty(field.getAnnotation(Column.class).name())) {
Column annotation = field.getAnnotation(Column.class);
sql.SELECT(annotation.name());
} else {
sql.SELECT(field.getName());
}
}
if (null != entity) {
for (int i = 0; i < fields.length; i++) {
Field field = fields[i];
field.setAccessible(true);
try {
Object value = field.get(entity);
if(null != value){
String col = getColName(field);
sql.WHERE(col + "=#{" + field.getName() + "}");
}
} catch (IllegalArgumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
for (Field field : orderby) {
if(field.getDeclaringClass().equals(entity.getClass())){
sql.ORDER_BY(getColName(field));
}
}
System.out.println(sql);
return sql.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy