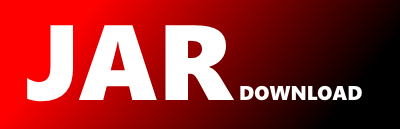
club.chlab.mybatis.provider.UpdateEntityProvider Maven / Gradle / Ivy
The newest version!
package club.chlab.mybatis.provider;
import java.lang.reflect.Field;
import org.apache.ibatis.jdbc.SQL;
import club.chlab.mybatis.annotations.Column;
import club.chlab.mybatis.annotations.Table;
import club.chlab.mybatis.exception.NoPrimaryKeyException;
/**
* mybatis update entity provider
* @author jch
*
*/
public class UpdateEntityProvider extends BaseProvider{
public String update(Object entity) throws Exception{
Class> cls = entity.getClass();
SQL sql = new SQL();
if(cls.isAnnotationPresent(Table.class) && !isEmpty(cls.getAnnotation(Table.class).name())){
Table annotation = cls.getAnnotation(Table.class);
sql.UPDATE(annotation.name());
}
else{
sql.UPDATE(cls.getSimpleName());
}
Field[] fields = cls.getDeclaredFields();
if(null != entity){
boolean primary = false;
for (int i = 0; i < fields.length; i++) {
Field field = fields[i];
field.setAccessible(true);
try {
Object value = field.get(entity);
if(null != value){
Column annotation = field.getAnnotation(Column.class);
String col = null == annotation || isEmpty(annotation.name()) ? field.getName() : annotation.name();
String val = "=#{"+field.getName()+"}";
if(null != annotation && annotation.primary()){
sql.WHERE(col+"=#{"+field.getName()+"}");
primary = true;
}
else{
sql.SET(col+val);
}
}
} catch (IllegalArgumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if(!primary){
throw new NoPrimaryKeyException();
}
}
System.out.println(sql);
return sql.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy