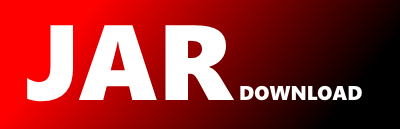
com.hs.gpxparser.modal.GPX Maven / Gradle / Ivy
package com.hs.gpxparser.modal;
import java.util.HashMap;
import java.util.HashSet;
/**
* This class holds gpx information from a <gpx> node.
*
* GPX specification for this tag:
*
*
* <gpx version="1.1" creator=""xsd:string [1]">
* <metadata> xsd:string </metadata> [0..1]
* <wpt> xsd:string </wpt> [0..1]
* <rte> xsd:string </rte> [0..1]
* <trk> xsd:string </trk> [0..1]
* <extensions> extensionsType </extensions> [0..1]
* </gpx>
*
*/
public class GPX extends Extension {
// Attributes
// TFE, 20180201: support multiple xmlns attributes
private HashMap xmlns;
private String creator;
private String version = "1.1";
// Nodes
private Metadata metadata;
private HashSet routes;
private HashSet
© 2015 - 2025 Weber Informatics LLC | Privacy Policy