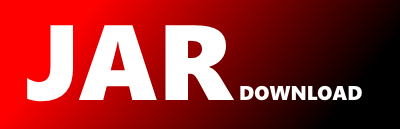
club.zhcs.lina.starter.exception.GlobalExceptionHandler Maven / Gradle / Ivy
package club.zhcs.lina.starter.exception;
import org.nutz.lang.Strings;
import org.nutz.log.Log;
import org.nutz.log.Logs;
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.MethodArgumentNotValidException;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.bind.annotation.RestControllerAdvice;
import club.zhcs.lina.auth.AuthenticationException;
import club.zhcs.lina.auth.AuthorizationException;
import club.zhcs.lina.starter.exception.event.ExceptionEvent;
import jakarta.validation.ValidationException;
import lombok.RequiredArgsConstructor;
/**
*
* @author Kerbores([email protected])
*
*/
@RestController
@RestControllerAdvice
@RequiredArgsConstructor
public class GlobalExceptionHandler {
private final ApplicationEventPublisher applicationEventPublisher;
Log log = Logs.get();
@ExceptionHandler(value = Exception.class)
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR)
public GlobalError defaultErrorHandler(Exception e) {
log.error(e);
applicationEventPublisher.publishEvent(ExceptionEvent.build(e));
return GlobalError.builder()
.code(HttpStatus.INTERNAL_SERVER_ERROR.value())
.message("服务器发生错误,请稍后再试!")
.build();
}
@ExceptionHandler(value = BizException.class)
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR)
public GlobalError bizExceptionHandler(Exception e) {
log.error(e);
return GlobalError.builder()
.code(HttpStatus.INTERNAL_SERVER_ERROR.value())
.message(e.getMessage())
.build();
}
@ExceptionHandler(ValidationException.class)
@ResponseStatus(HttpStatus.BAD_REQUEST)
public GlobalError handle(ValidationException e) {
log.error(e);
return GlobalError.builder()
.code(HttpStatus.BAD_REQUEST.value())
.message("请求参数校验不通过")
.build();
}
@ExceptionHandler(value = MethodArgumentNotValidException.class)
@ResponseStatus(HttpStatus.BAD_REQUEST)
public GlobalError validate(MethodArgumentNotValidException e) {
log.error(e);
return GlobalError.builder()
.code(HttpStatus.BAD_REQUEST.value())
.message("请求参数不正确")
.build();
}
@ExceptionHandler(value = AuthenticationException.class)
@ResponseStatus(HttpStatus.UNAUTHORIZED)
public GlobalError authentication(AuthenticationException e) {
log.error(e);
return GlobalError.builder()
.code(HttpStatus.UNAUTHORIZED.value())
.message(Strings.isBlank(e.getMessage()) ? "身份认证失败!" : e.getMessage())
.build();
}
@ExceptionHandler(value = AuthorizationException.class)
@ResponseStatus(HttpStatus.FORBIDDEN)
public GlobalError authorization(AuthorizationException e) {
log.error(e);
return GlobalError.builder()
.code(HttpStatus.FORBIDDEN.value())
.message(Strings.isBlank(e.getMessage()) ? "没有权限进行操作!" : e.getMessage())
.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy