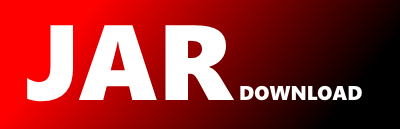
club.zhcs.lina.web.response.GlobalResponseHandler Maven / Gradle / Ivy
The newest version!
package club.zhcs.lina.web.response;
import java.util.List;
import java.util.regex.Pattern;
import org.nutz.lang.Lang;
import org.springframework.core.MethodParameter;
import org.springframework.http.MediaType;
import org.springframework.http.converter.HttpMessageConverter;
import org.springframework.http.server.ServerHttpRequest;
import org.springframework.http.server.ServerHttpResponse;
import org.springframework.lang.NonNull;
import org.springframework.lang.Nullable;
import org.springframework.web.bind.annotation.RestControllerAdvice;
import org.springframework.web.servlet.mvc.method.annotation.ResponseBodyAdvice;
/**
*
* @author Kerbores([email protected])
*
*/
@RestControllerAdvice
public class GlobalResponseHandler implements ResponseBodyAdvice
© 2015 - 2025 Weber Informatics LLC | Privacy Policy