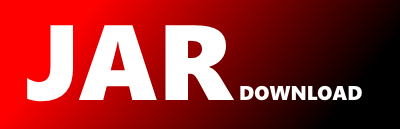
cn.coder.jdbc.support.JSql Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdbc Show documentation
Show all versions of jdbc Show documentation
A simple, light Java ORM framework.
package cn.coder.jdbc.support;
import java.util.ArrayList;
import java.util.List;
/**
* 复杂SQL构造类
*
* @author YYDF 2019-07-29
*/
public final class JSql {
private final List temps;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy