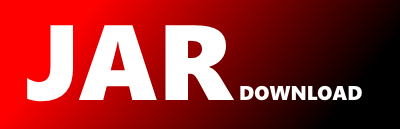
cn.acyou.leo.framework.push.umeng.PushClient Maven / Gradle / Ivy
package cn.acyou.leo.framework.push.umeng;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.codec.digest.DigestUtils;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClients;
import org.json.JSONObject;
import java.io.BufferedReader;
import java.io.InputStreamReader;
@Slf4j
public class PushClient {
// The user agent
protected final String USER_AGENT = "Mozilla/5.0";
// This object is used for sending the post request to Umeng
protected HttpClient client = HttpClients.createDefault();
// The host
protected static final String host = "http://msg.umeng.com";
// The upload path
protected static final String uploadPath = "/upload";
// The post path
protected static final String postPath = "/api/send";
public boolean send(UmengNotification msg) throws Exception {
String timestamp = Integer.toString((int) (System.currentTimeMillis() / 1000));
msg.setPredefinedKeyValue("timestamp", timestamp);
String url = host + postPath;
String postBody = msg.getPostBody();
String sign = DigestUtils.md5Hex(("POST" + url + postBody + msg.getAppMasterSecret()).getBytes("utf8"));
url = url + "?sign=" + sign;
HttpPost post = new HttpPost(url);
post.setHeader("User-Agent", USER_AGENT);
StringEntity se = new StringEntity(postBody, "UTF-8");
post.setEntity(se);
// Send the post request and get the response
HttpResponse response = client.execute(post);
int status = response.getStatusLine().getStatusCode();
log.info("Response Code : " + status);
BufferedReader rd = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
StringBuffer result = new StringBuffer();
String line = "";
while ((line = rd.readLine()) != null) {
result.append(line);
}
log.info("Send res:{}", result.toString());
if (status == 200) {
log.info("Notification sent successfully.");
} else {
log.info("Failed to send the notification!");
}
return true;
}
/**
* Upload file with device_tokens to Umeng
*
* @param appkey
* @param appMasterSecret
* @param contents
* @return
* @throws Exception
*/
public String uploadContents(String appkey, String appMasterSecret, String contents) throws Exception {
// Construct the json string
JSONObject uploadJson = new JSONObject();
uploadJson.put("appkey", appkey);
String timestamp = Integer.toString((int) (System.currentTimeMillis() / 1000));
uploadJson.put("timestamp", timestamp);
uploadJson.put("content", contents);
// Construct the request
String url = host + uploadPath;
String postBody = uploadJson.toString();
String sign = DigestUtils.md5Hex(("POST" + url + postBody + appMasterSecret).getBytes("utf8"));
url = url + "?sign=" + sign;
HttpPost post = new HttpPost(url);
post.setHeader("User-Agent", USER_AGENT);
StringEntity se = new StringEntity(postBody, "UTF-8");
post.setEntity(se);
// Send the post request and get the response
HttpResponse response = client.execute(post);
log.info("Response Code : " + response.getStatusLine().getStatusCode());
BufferedReader rd = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
StringBuffer result = new StringBuffer();
String line = "";
while ((line = rd.readLine()) != null) {
result.append(line);
}
log.info(result.toString());
// Decode response string and get file_id from it
JSONObject respJson = new JSONObject(result.toString());
String ret = respJson.getString("ret");
if (!ret.equals("SUCCESS")) {
throw new Exception("Failed to upload file");
}
JSONObject data = respJson.getJSONObject("data");
String fileId = data.getString("file_id");
// Set file_id into rootJson using setPredefinedKeyValue
return fileId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy