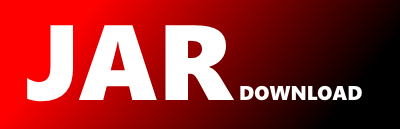
cn.allbs.hj212.core.MultipleCharMatch Maven / Gradle / Ivy
package cn.allbs.hj212.core;
import cn.allbs.hj212.lambda.RunnableWithThrowable;
import cn.allbs.hj212.lambda.SupplierWithThrowable;
import java.io.IOException;
import java.io.PushbackReader;
import java.util.Arrays;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Optional;
import java.util.function.Predicate;
import java.util.function.Supplier;
/**
* 功能:
*
* @author chenQi
*/
public class MultipleCharMatch implements ReaderMatch, ParentStream, char[]> {
private ParentStream parentStream;
private PushbackReader reader;
private int count;
private boolean alwaysRollBack = false;
private Map, SupplierWithThrowable, IOException>> map;
private MapEntryStepGenerator, SupplierWithThrowable, IOException>> generator;
public MultipleCharMatch(ParentStream parentStream, int count) {
this.parentStream = parentStream;
this.reader = parentStream.reader();
this.count = count;
this.map = new LinkedHashMap<>();
MapEntryStepGenerator.Builder, SupplierWithThrowable, IOException>> builder = MapEntryStepGenerator.builder();
this.generator = builder.consumer((k, v) -> map.put(k, v))
.keyDefault(() -> character -> false)
.keyMergeOperator(Predicate::or)
.valueMergeOperator((thisRunnable, runnable) -> () -> {
Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy