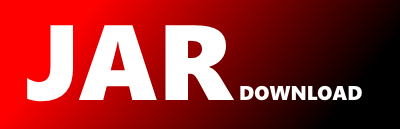
cn.allbs.hj212.format.T212Mapper Maven / Gradle / Ivy
package cn.allbs.hj212.format;
import cn.allbs.hj212.config.T212Configurator;
import cn.allbs.hj212.deser.*;
import cn.allbs.hj212.exception.T212FormatException;
import cn.allbs.hj212.feature.Feature;
import cn.allbs.hj212.feature.ParserFeature;
import cn.allbs.hj212.feature.SegmentParserFeature;
import cn.allbs.hj212.feature.VerifyFeature;
import cn.allbs.hj212.model.HjData;
import cn.allbs.hj212.ser.CpDataLevelMapDataSerializer;
import cn.allbs.hj212.ser.DataSerializer;
import cn.allbs.hj212.ser.PackLevelSerializer;
import cn.allbs.hj212.ser.T212Serializer;
import com.fasterxml.jackson.databind.ObjectMapper;
import javax.validation.Validator;
import java.io.*;
import java.lang.reflect.Type;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
import java.util.function.Supplier;
/**
* 功能: T212映射器
*
* @author chenQi
*/
public class T212Mapper {
private static T212Factory t212FactoryProtoType;
static {
try {
t212FactoryProtoType = new T212Factory();
//注册 反序列化器
t212FactoryProtoType.deserializerRegister(CpDataLevelMapDeserializer.class);
t212FactoryProtoType.deserializerRegister(DataLevelMapDeserializer.class);
t212FactoryProtoType.deserializerRegister(PackLevelDeserializer.class);
t212FactoryProtoType.deserializerRegister(Map.class, CpDataLevelMapDeserializer.class);
t212FactoryProtoType.deserializerRegister(HjData.class, DataDeserializer.class);
//默认 反序列化器
t212FactoryProtoType.deserializerRegister(Object.class, CpDataLevelMapDeserializer.class);
//注册 序列化器
t212FactoryProtoType.serializerRegister(PackLevelSerializer.class);
t212FactoryProtoType.serializerRegister(HjData.class, DataSerializer.class);
t212FactoryProtoType.serializerRegister(Map.class, CpDataLevelMapDataSerializer.class);
//没有默认 序列化器
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (InstantiationException e) {
e.printStackTrace();
}
}
private int verifyFeatures;
private int parserFeatures;
private T212Factory factory;
private T212Configurator configurator;
private Validator validator;
private ObjectMapper objectMapper;
public T212Mapper() {
this.factory = t212FactoryProtoType.copy();
this.configurator = new T212Configurator();
this.validator = factory.validator();
this.objectMapper = factory.objectMapper();
}
/*
/**********************************************************
/* Configuration
/**********************************************************
*/
private static final int SEGMENT_FEATURE_BIT_OFFSET = 8;
public T212Mapper enableDefaultParserFeatures() {
parserFeatures = Feature.collectFeatureDefaults(SegmentParserFeature.class);
parserFeatures = parserFeatures << SEGMENT_FEATURE_BIT_OFFSET;
parserFeatures = parserFeatures | Feature.collectFeatureDefaults(ParserFeature.class);
return this;
}
public T212Mapper enableDefaultVerifyFeatures() {
verifyFeatures = verifyFeatures | Feature.collectFeatureDefaults(VerifyFeature.class);
return this;
}
public T212Mapper enable(SegmentParserFeature feature) {
parserFeatures = parserFeatures | feature.getMask() << SEGMENT_FEATURE_BIT_OFFSET;
return this;
}
public T212Mapper enable(ParserFeature feature) {
parserFeatures = parserFeatures | feature.getMask();
return this;
}
public T212Mapper enable(VerifyFeature feature) {
verifyFeatures = verifyFeatures | feature.getMask();
return this;
}
public T212Mapper disable(SegmentParserFeature feature) {
parserFeatures = parserFeatures & ~(feature.getMask() << SEGMENT_FEATURE_BIT_OFFSET);
return this;
}
public T212Mapper disable(ParserFeature feature) {
parserFeatures = parserFeatures & ~feature.getMask();
return this;
}
public T212Mapper disable(VerifyFeature feature) {
verifyFeatures = verifyFeatures & ~feature.getMask();
return this;
}
public T212Mapper configurator(T212Configurator configurator) {
this.configurator = configurator;
return this;
}
public ObjectMapper objectMapper() {
return this.objectMapper;
}
private T212Mapper applyConfigurator() {
configurator.setSegmentParserFeature(this.parserFeatures >> SEGMENT_FEATURE_BIT_OFFSET);
configurator.setParserFeature(this.parserFeatures & 0x00FF);
configurator.setVerifyFeature(this.verifyFeatures);
configurator.setValidator(this.validator);
configurator.setObjectMapper(this.objectMapper);
factory.setConfigurator(configurator);
return this;
}
public T readValue(InputStream is, Class value) throws IOException, T212FormatException {
applyConfigurator();
return _readValueAndClose(factory.parser(is), value);
}
public T readValue(byte[] bytes, Class value) throws IOException, T212FormatException {
applyConfigurator();
return _readValueAndClose(factory.parser(bytes), value);
}
public T readValue(Reader reader, Class value) throws IOException, T212FormatException {
applyConfigurator();
return _readValueAndClose(factory.parser(reader), value);
}
public T readValue(String data, Class value) throws IOException, T212FormatException {
applyConfigurator();
return _readValueAndClose(factory.parser(data), value);
}
private T _readValueAndClose(T212Parser parser, Class value) throws IOException, T212FormatException {
T212Deserializer deserializer = factory.deserializerFor(value);
try (T212Parser p = parser) {
return deserializer.deserialize(p);
} catch (RuntimeException e) {
throw new T212FormatException("Runtime error", e);
}
}
private T _readValueAndClose(T212Parser parser, Type type, Class value) throws IOException, T212FormatException {
T212Deserializer deserializer = factory.deserializerFor(type, value);
try (T212Parser p = parser) {
return deserializer.deserialize(p);
} catch (RuntimeException e) {
throw new T212FormatException("Runtime error", e);
}
}
public void writeValueAsStream(T value, Class type, OutputStream outputStream) throws IOException, T212FormatException {
applyConfigurator();
_writeValueAndClose(factory.generator(outputStream), value, type);
}
public void writeValueAsWriter(T value, Class type, Writer writer) throws IOException, T212FormatException {
applyConfigurator();
_writeValueAndClose(factory.generator(writer), value, type);
}
private void _writeValueAndClose(T212Generator generator, T value, Class type) throws IOException, T212FormatException {
T212Serializer serializer = factory.serializerFor(type);
try (T212Generator g = generator) {
serializer.serialize(g, value);
} catch (RuntimeException e) {
throw new T212FormatException("Runtime error", e);
}
}
private static Supplier getMapGenericType() {
return () -> new Map() {
@Override
public int size() {
return 0;
}
@Override
public boolean isEmpty() {
return false;
}
@Override
public boolean containsKey(Object key) {
return false;
}
@Override
public boolean containsValue(Object value) {
return false;
}
@Override
public String get(Object key) {
return null;
}
@Override
public String put(String key, String value) {
return null;
}
@Override
public String remove(Object key) {
return null;
}
@Override
public void putAll(Map extends String, ? extends String> m) {
}
@Override
public void clear() {
}
@Override
public Set keySet() {
return null;
}
@Override
public Collection values() {
return null;
}
@Override
public Set> entrySet() {
return null;
}
}.getClass().getGenericInterfaces()[0];
}
private static Supplier getDeepMapGenericType() {
return () -> new Map() {
@Override
public int size() {
return 0;
}
@Override
public boolean isEmpty() {
return false;
}
@Override
public boolean containsKey(Object key) {
return false;
}
@Override
public boolean containsValue(Object value) {
return false;
}
@Override
public String get(Object key) {
return null;
}
@Override
public String put(String key, Object value) {
return null;
}
@Override
public String remove(Object key) {
return null;
}
@Override
public void putAll(Map extends String, ? extends Object> m) {
}
@Override
public void clear() {
}
@Override
public Set keySet() {
return null;
}
@Override
public Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy