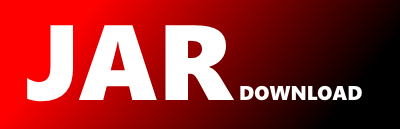
cn.allbs.hj212.validator.clazz.FieldValidator Maven / Gradle / Ivy
package cn.allbs.hj212.validator.clazz;
import cn.allbs.hj212.exception.T212FormatException;
import javax.validation.ConstraintValidator;
import javax.validation.ConstraintValidatorContext;
import javax.validation.ConstraintViolation;
import java.lang.annotation.Annotation;
import java.util.*;
import java.util.function.BiConsumer;
import java.util.function.BinaryOperator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collector;
import java.util.stream.Collectors;
/**
* @author ChenQi
*/
public abstract class FieldValidator
implements ConstraintValidator {
protected String field;
private AF af;
private ConstraintValidator constraintValidator;
public FieldValidator(ConstraintValidator constraintValidator) {
this.constraintValidator = constraintValidator;
}
@Override
public void initialize(A a) {
this.field = getField(a);
this.af = getAnnotation(a);
constraintValidator.initialize(af);
}
@Override
public boolean isValid(V value, ConstraintValidatorContext constraintValidatorContext) {
FV fv = getFieldValue(value, field);
boolean result = constraintValidator.isValid(fv, constraintValidatorContext);
if (!result) {
constraintValidatorContext.disableDefaultConstraintViolation();
constraintValidatorContext
.buildConstraintViolationWithTemplate(getFieldMessage(af))
.addPropertyNode(field).addConstraintViolation();
}
return result;
}
public abstract String getField(A a);
public abstract AF getAnnotation(A a);
public abstract FV getFieldValue(V value, String field);
public abstract String getFieldMessage(AF value);
public static void create_format_exception(Set extends ConstraintViolation> constraintViolationSet, Object result) throws T212FormatException {
Map typePropertys = constraintViolationSet
.stream()
.collect(Collectors.groupingBy(
ConstraintViolation::getMessage,
new Collector() {
@Override
public Supplier supplier() {
return () -> new StringJoiner(",", "", "");
}
@Override
public BiConsumer accumulator() {
return (s, cv) -> s.add("'" + cv.getPropertyPath() + "'");
}
@Override
public BinaryOperator combiner() {
return StringJoiner::merge;
}
@Override
public Function finisher() {
return StringJoiner::toString;
}
@Override
public Set characteristics() {
return Collections.emptySet();
}
}
));
String msg = typePropertys.entrySet()
.stream()
.map(kv -> "\t"
+ kv.getKey()
+ " -> "
+ kv.getValue())
.collect(Collectors.joining("\n"));
throw new T212FormatException("Validate error\n" + msg)
.withResult(result);
}
public static void create_format_exception2(Set extends ConstraintViolation> constraintViolationSet) throws T212FormatException {
Map> typeCVs = constraintViolationSet
.stream()
.collect(Collectors.groupingBy(
ConstraintViolation::getMessage,
Collectors.toList())
);
Map typePropertys = typeCVs.entrySet()
.stream()
.map(kv -> {
String pps = kv.getValue()
.stream()
.map(cv -> "'" + cv.getPropertyPath() + "'")
.collect(Collectors.joining(","));
return new AbstractMap.SimpleEntry<>(kv.getKey(), pps);
})
.collect(Collectors.toMap(AbstractMap.SimpleEntry::getKey, AbstractMap.SimpleEntry::getValue));
String msg = typePropertys.entrySet()
.stream()
.map(kv -> "\t"
+ kv.getKey()
+ " -> "
+ kv.getValue())
.collect(Collectors.joining("\n"));
throw new T212FormatException("Validate error\n" + msg);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy