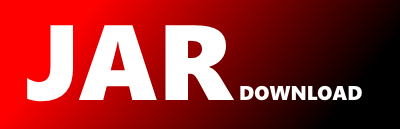
cn.apiclub.captcha.audio.producer.RandomNumberVoiceProducer Maven / Gradle / Ivy
The newest version!
package cn.apiclub.captcha.audio.producer;
import java.security.SecureRandom;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Random;
import cn.apiclub.captcha.audio.Sample;
import cn.apiclub.captcha.util.FileUtil;
/**
*
* {@link VoiceProducer} which generates a vocalization for a given number,
* randomly selecting from a list of voices. The default voices are located in
* the jar in the sounds/en/numbers
directory, and have filenames
* with a format of num-voice.wav, e.g.:
* sounds/en/numbers/1-alex.wav
.
*
*
* @author James Childers
*
*/
public class RandomNumberVoiceProducer implements VoiceProducer {
private static final Random RAND = new SecureRandom();
private static final String[] DEFAULT_VOICES = { "alex", "bruce", "fred",
"ralph", "kathy", "vicki", "victoria" };
private static final Map DEFAULT_VOICES_MAP;
static {
DEFAULT_VOICES_MAP = new HashMap();
String[] files_for_num;
StringBuilder sb;
for (int i = 0; i < 10; i++) {
files_for_num = new String[DEFAULT_VOICES.length];
for (int j = 0; j < files_for_num.length; j ++) {
sb = new StringBuilder("/sounds/en/numbers/");
sb.append(i);
sb.append("-");
sb.append(DEFAULT_VOICES[j]);
sb.append(".wav");
files_for_num[j] = sb.toString();
}
DEFAULT_VOICES_MAP.put(i, files_for_num);
}
}
private final Map _voices;
public RandomNumberVoiceProducer() {
this(DEFAULT_VOICES_MAP);
}
/**
* Creates a RandomNumberVoiceProducer
for the given
* voices
, a map of numbers to their corresponding filenames.
* Conceptually the map must look like the following:
*
*
* {1 => ["/my_sounds/1-quiet.wav", "/my_sounds/1-loud.wav"],
* 2 => ["/my_sounds/2-quiet.wav", "/my_sounds/2-loud.wav"]}
*
*
* @param voices
*/
public RandomNumberVoiceProducer(Map voices) {
_voices = voices;
}
public Map getVoices() {
return Collections.unmodifiableMap(_voices);
}
@Override public final Sample getVocalization(char num) {
try {
Integer.parseInt(num + "");
} catch (NumberFormatException e) {
throw new IllegalArgumentException(
"Expected to be a number, got '" + num + "' instead.",
e);
}
int idx = Integer.parseInt(num + "");
String[] files = _voices.get(idx);
String filename = files[RAND.nextInt(files.length)];
return FileUtil.readSample(filename);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy