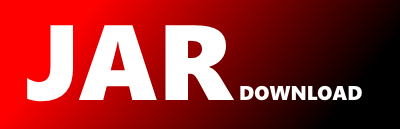
cn.authing.core.mgmt.RolesManagementClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-core Show documentation
Show all versions of java-core Show documentation
java backend sdk for authing
package cn.authing.core.mgmt
import cn.authing.core.Utils
import cn.authing.core.graphql.GraphQLCall
import cn.authing.core.graphql.GraphQLResponse
import cn.authing.core.http.HttpCall
import cn.authing.core.types.*
import com.google.gson.Gson
import com.google.gson.GsonBuilder
import com.google.gson.reflect.TypeToken
import java.util.*
/**
* 角色管理类
*/
class RolesManagementClient(private val client: ManagementClient) {
/**
* 获取角色列表
*/
@JvmOverloads
fun list(
page: Int? = null,
limit: Int? = null,
sortBy: SortByEnum? = null,
namespace: String? = null
): HttpCall, PaginatedRoles> {
val param = RolesParam(namespace, page, limit, sortBy)
return list(param)
}
/**
* 获取角色列表
*/
fun list(param: RolesParam): HttpCall, PaginatedRoles> {
var url = "${client.host}/api/v2/roles"
url += if (param.page != null) "?page=${param.page}" else "?page=1"
url += if (param.limit != null) "&limit=${param.limit}" else "&limit=10"
url += if (param.sortBy != null) "&sortBy=${param.sortBy}" else ""
url += if (param.namespace != null) "&namespace=${param.namespace}" else ""
return client.createHttpGetCall(
url,
object : TypeToken> () {}
) {
it.data
}
}
/**
* 创建角色
*/
@JvmOverloads
fun create(
code: String,
description: String? = null,
parent: String? = null,
namespace: String? = null
): HttpCall, Role> {
val param = CreateRoleParam(namespace, code, description, parent)
return create(param)
}
/**
* 创建角色
*/
fun create(param: CreateRoleParam): HttpCall, Role> {
return client.createHttpPostCall(
"${client.host}/api/v2/roles",
GsonBuilder().create().toJson(param),
object : TypeToken> () {}
) {
it.data
}
}
/**
* 角色详情
*/
@Deprecated("use findByCode", ReplaceWith("this.findByCode(param)"))
fun detail(code: String): GraphQLCall {
val param = RoleParam(code)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 获取角色详情
*/
fun findByCode(param: RoleParam): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 修改角色
*/
@JvmOverloads
fun update(
code: String,
description: String? = null,
newCode: String? = null
): GraphQLCall {
val param = UpdateRoleParam(code, description, newCode)
return update(param)
}
/**
* 修改角色
*/
fun update(param: UpdateRoleParam): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 删除角色
*/
fun delete(code: String): GraphQLCall {
val param = DeleteRoleParam(code)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 删除角色
*/
fun delete(param: DeleteRoleParam): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 批量删除角色
*/
fun deleteMany(codeList: List): GraphQLCall {
val param = DeleteRolesParam(codeList)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 批量删除角色
*/
fun deleteMany(param: DeleteRolesParam): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 获取角色用户列表
*/
fun listUsers(code: String): GraphQLCall {
val param = RoleWithUsersParam(code)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result.users
}
}
/**
* 获取角色用户列表
*/
fun listUsers(param: RoleWithUsersParam): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result.users
}
}
/**
* 添加用户
*/
fun addUsers(code: String, userIds: List): GraphQLCall {
val param = AssignRoleParam().withUserIds(userIds).withRoleCode(code)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 添加用户
*/
fun addUsers(param: AssignRoleParam): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 移除用户
*/
fun removeUsers(code: String, userIds: List): GraphQLCall {
val param = RevokeRoleParam().withRoleCode(code).withUserIds(userIds)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 移除用户
*/
fun removeUsers(param: RevokeRoleParam): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 获取角色策略列表
*/
@JvmOverloads
fun listPolicies(
code: String,
page: Int? = null,
limit: Int? = null,
namespace: String? = null,
targetIdentifier: String? = null
): GraphQLCall {
val param =
PolicyAssignmentsParam(namespace, code, PolicyAssignmentTargetType.ROLE, targetIdentifier, page, limit)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 给角色授权策略
*/
fun addPolicies(
code: String,
policies: List
): GraphQLCall {
val param =
AddPolicyAssignmentsParam(policies, PolicyAssignmentTargetType.ROLE).withTargetIdentifiers(listOf(code))
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 角色移除策略
*/
fun removePolicies(
code: String,
policies: List
): GraphQLCall {
val param =
RemovePolicyAssignmentsParam(policies, PolicyAssignmentTargetType.ROLE).withTargetIdentifiers(listOf(code))
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 获取角色被授权的所有资源
*/
fun listAuthorizedResources(
param: ListRoleAuthorizedResourcesParam
): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}
) { it.result.authorizedResources }
}
/**
* 获取某个角色扩展字段列表
*/
fun getUdfValue(roleCode: String): GraphQLCall> {
val param = UdvParam(UdfTargetType.ROLE, roleCode)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
convertUdvToKeyValuePair(it.result)
}
}
/**
* 获取多个角色扩展字段列表
*/
fun getUdfValueBatch(roleCodes: List): GraphQLCall>> {
if (roleCodes.isEmpty()) {
throw Exception("userIds can't be null")
}
val param = UdfValueBatchParam(UdfTargetType.ROLE, roleCodes)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
val hashtable = Hashtable>()
it.result.map { hashtable.put(it.targetId, convertUdvToKeyValuePair(it.data)) }
hashtable
}
}
/**
* 设置某个角色扩展字段列表
*/
fun setUdfValue(
roleCode: String,
data: Map
): HttpCall>, List> {
val params = RestSetUdfValueParams(UdfTargetType.ROLE, roleCode, data)
return client.createHttpPostCall(
"${client.host}/api/v2/udvs",
GsonBuilder().create().toJson(params),
object : TypeToken>> () {}
) {
it.data
}
}
/**
* 设置某个角色扩展字段列表
*/
fun setUdfValue(
roleCode: String,
key: String,
value: String
): HttpCall>, List> {
val map: MutableMap = HashMap()
map[key] = value
val param = RestSetUdfValueParams(UdfTargetType.ROLE, roleCode, map)
return client.createHttpPostCall(
"${client.host}/api/v2/udvs",
GsonBuilder().create().toJson(param),
object : TypeToken>> () {}
) {
it.data
}
}
/**
* 设置多个角色扩展字段列表
*/
fun setUdfValueBatch(input: List): GraphQLCall> {
if (input.isEmpty()) {
throw Exception("empty input list")
}
val param = SetUdfValueBatchParam(UdfTargetType.ROLE,
input.flatMap { item ->
item.data.map { SetUdfValueBatchInput(item.roleCode, it.key, Gson().toJson(it.value)) }
}
)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {})
{
it.result
}
}
/**
* 删除用户的扩展字段
*/
fun removeUdfValue(roleCode: String, key: String): GraphQLCall> {
val param = RemoveUdvParam(UdfTargetType.ROLE, roleCode, key)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 获取某个角色某个扩展字段
*/
fun getSpecificUdfValue(roleCode: String, udfKey: String) : GraphQLCall {
val param = UdvParam(UdfTargetType.ROLE, roleCode)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
val iterator = it.result.iterator()
var res: UserDefinedData? = null
while (iterator.hasNext()) {
val next = iterator.next()
if (next.key == udfKey) {
res = next
}
}
res
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy