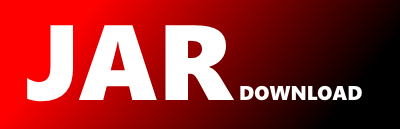
cn.authing.core.mgmt.TenantManagementClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-core Show documentation
Show all versions of java-core Show documentation
java backend sdk for authing
package cn.authing.core.mgmt
import cn.authing.core.http.HttpCall
import cn.authing.core.types.*
import com.google.gson.Gson
import com.google.gson.GsonBuilder
import com.google.gson.reflect.TypeToken
class TenantManagementClient(private val client: ManagementClient) {
/**
* 创建租户
*/
fun create(
options: CreateTenantParams
): HttpCall, CreateTenantResponse> {
return client.createHttpPostCall(
"${client.host}/api/v2/tenant",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it.data
}
}
/**
* 根据租户 ID 查询租户
*/
fun details(tenantId: String): HttpCall, TenantDetail> {
return client.createHttpGetCall(
"${client.host}/api/v2/tenant/${tenantId}",
object : TypeToken>() {}) {
it.data
}
}
/**
* 获取租户列表
*/
fun list(page: Number, limit: Number): HttpCall, PaginatedTenants> {
return client.createHttpGetCall(
"${client.host}/api/v2/tenants?page=${page}&limit=${limit}",
object : TypeToken>() {}) {
it.data
}
}
/**
* 修改租户信息
*/
fun update(
tenantId: String,
options: UpdateTenantParams
): HttpCall, Boolean> {
return client.createHttpPostCall(
"${client.host}/api/v2/tenant/${tenantId}",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it.data
}
}
/**
* 刪除租戶
*/
fun delete(
tenantId: String
): HttpCall, Boolean> {
val url = "${this.client.host}/api/v2/tenant/${tenantId}"
return client.createHttpDeleteCall(
url,
object : TypeToken>() {}
) {
it.code == 200
it.message == "删除租户成功"
}
}
/**
* 配置租户品牌化
*/
fun config(
tenantId: String,
options: ConfigSsoPageCustomizationSetting
): HttpCall, Boolean> {
return client.createHttpPostCall(
"${client.host}/api/v2/tenant/${tenantId}",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it.data
}
}
/**
* 添加租户成员
*/
fun addMembers(
tenantId: String,
options: UserTenantIdList
): HttpCall, CreateTenantMemberResponse> {
return client.createHttpPostCall(
"${client.host}/api/v2/tenant/${tenantId}/user",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it.data
}
}
/**
* 删除租户成员
*/
fun removeMembers(tenantId: String, userId: String): HttpCall {
return client.createHttpDeleteCall(
"${client.host}/api/v2/tenant/${tenantId}/user?userId=${userId}",
object : TypeToken() {}) {
it
}
}
/**
* 更新租户成员
*/
fun updateTenantMember(
tenantId: String,
userId: String,
options: UpdateTenantMemberParam
): HttpCall, CreateIdpResponse> {
return client.createHttpPutCall(
"${client.host}/api/v2/tenant/${tenantId}/${userId}",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it.data
}
}
/**
* 获取租户成员列表
*/
fun memeber(
tenantId: String,
page: Number,
limit: Number
): HttpCall, PaginatedTenants> {
return client.createHttpGetCall(
"${client.host}/api/v2/tenant/${tenantId}/users?page=${page}&limit=${limit}",
object : TypeToken>() {}) {
it.data
}
}
/**
* 创建身份源
*/
fun createExtIdp(options: CreateIdpParam): HttpCall, CreateIdpResponse> {
return client.createHttpPostCall(
"${client.host}/api/v2/extIdp",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it.data
}
}
/**
* 更新身份源
*/
fun updateExtIdp(
extIdpId: String,
options: UpdateIdpParam
): HttpCall, CreateIdpResponse> {
return client.createHttpPutCall(
"${client.host}/api/v2/extIdp/${extIdpId}",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it.data
}
}
/**
* 删除身份源
*/
fun deleteExtIdp(extIdpId: String): HttpCall {
return client.createHttpDeleteCall(
"${client.host}/api/v2/extIdp/${extIdpId}",
object : TypeToken() {}) {
it
}
}
/**
* 获取身份源详细信息
*/
fun extIdpDetail(extIdpId: String): HttpCall, CreateIdpResponse> {
return client.createHttpGetCall(
"${client.host}/api/v2/extIdp/${extIdpId}",
object : TypeToken>() {}) {
it.data
}
}
/**
* 获取身份源列表
*/
fun listExtIdp(tenantId: String): HttpCall>, List> {
return client.createHttpGetCall(
"${client.host}/api/v2/extIdp?tenantId=${tenantId}",
object : TypeToken>>() {}) {
it.data
}
}
fun listExtIdp(
tenantId: String?,
type: ExtIdpListTypeEnum,
appId: String?
): HttpCall>, List> {
return client.createHttpGetCall(
"${client.host}/api/v2/extIdp?tenantId=${tenantId}&type=${type}&appId=${appId}",
object : TypeToken>>() {}) {
it.data
}
}
/**
*创建身份源连接
*/
fun createExtIdpConnection(options: CreatIdpConnParam): HttpCall, CreateIdpConnResponse> {
return client.createHttpPostCall(
"${client.host}/api/v2/extIdpConn",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it.data
}
}
/**
* 更新身份源连接
*/
fun updateExtIdpConnection(
extIdpConnectionId: String,
options: UpdateIdpConnParm
): HttpCall {
return client.createHttpPutCall(
"${client.host}/api/v2/extIdpConn/${extIdpConnectionId}",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
/**
* 删除身份源连接
*/
fun deleteExtIdpConnection(extIdpConnectionId: String): HttpCall {
return client.createHttpDeleteCall(
"${client.host}/api/v2/extIdpConn/${extIdpConnectionId}",
object : TypeToken() {}) {
it
}
}
/**
*检查连接唯一标识是否冲突
*/
fun checkExtIdpConnectionIdentifierUnique(options: CheckExtIdpConnectionIdentifierUnique): HttpCall {
return client.createHttpPostCall(
"${client.host}/api/v2/check/extIdpConn/identifier",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
/**
* 开关身份源连接
*/
fun changeExtIdpConnectionState(
extIdpConnectionId: String,
options: ConnState
): HttpCall {
return client.createHttpPutCall(
"${client.host}/api/v2/extIdpConn/${extIdpConnectionId}/state",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
/**
* 批量开关身份源连接
*/
fun batchChangeExtIdpConnectionState(
extIdpConnectionId: String,
options: ConnState
): HttpCall {
return client.createHttpPutCall(
"${client.host}/api/v2/extIdp/${extIdpConnectionId}/connState",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
/**
* 设置租户管理员
*/
fun setTanentAdmin(tenantId: String, options: UserTenantIdList): HttpCall {
return client.createHttpPutCall(
"${client.host}/api/v2/tenant/${tenantId}/admin",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
/**
* 取消租户管理员
*/
fun deleteTanentAdmin(tenantId: String, userId: String): HttpCall {
return client.createHttpDeleteCall(
"${client.host}/api/v2/tenant/${tenantId}/admin/${userId}",
object : TypeToken() {}) {
it
}
}
// /**
// * 用户池管理员给租户管理员授权资源
// */
// fun userPoolAdminAddTanentAdminAuthorizeResources(options:UserPoolAdminToTanentAdminAuthorizeResourcesParam): HttpCall {
// return client.createHttpPostCall(
// "${client.host}/api/v2/acl/tenant/authorize-resources-for-tenant",
// GsonBuilder().create().toJson(options),
// object : TypeToken() {}
// ) {
// it
// }
// }
//
// /**
// * 用户池管理员给租户成员撤销资源
// */
// fun revokeResources(options:UserPoolAdminToTanentAdminAuthorizeResourcesParam): HttpCall {
// return client.createHttpPostCall(
// "${client.host}/api/v2/acl/tenant/revoke-resources-for-tenant",
// GsonBuilder().create().toJson(options),
// object : TypeToken() {}
// ) {
// it
// }
// }
//
// /**
// * 用户池管理员获取租户管理员被授权的业务资源
// */
// fun userPoolAdminGetTenantAdminResourceList(
// tenantId: String,
// userId:String,
// resourceType:String): HttpCall, UserPoolAdminGetTenantAdminResourceList> {
// return client.createHttpGetCall(
// "${client.host}/api/v2/acl/tenant/tenant-authorized-resources?tenant_id=${tenantId}&user_id=${userId}&resource_type=${resourceType}",
// object : TypeToken>() {}) {
// it.data
// }
// }
//
// /**
// * 租户管理员给租户成员授权资源
// */
// fun tanentAdminAddTanentMemberAuthorizeResources(options:TanentAdminAddTanentMemberAuthorizeResourcesParam): HttpCall {
// return client.createHttpPostCall(
// "${client.host}/api/v2/acl/tenant/authorize-resources",
// GsonBuilder().create().toJson(options),
// object : TypeToken() {}
// ) {
// it
// }
// }
//
// /**
// * 租户管理员给租户成员取消授权资源
// */
// fun tenantAdminRevokeResources(options:TanentAdminAddTanentMemberAuthorizeResourcesParam): HttpCall {
// return client.createHttpPostCall(
// "${client.host}/api/v2/acl/tenant/revoke-resources",
// GsonBuilder().create().toJson(options),
// object : TypeToken() {}
// ) {
// it
// }
// }
//
// /**
// * 租户管理员获取租户成员被授权的资源列表
// */
// fun tenantAdminGetTenantMemberResourceList(
// userId:String,
// resourceType:String): HttpCall, UserPoolAdminGetTenantAdminResourceList> {
// return client.createHttpGetCall(
// "${client.host}/api/v2/acl/tenant/authorized-resources?user_id=${userId}&resource_type=${resourceType}",
// object : TypeToken>() {}) {
// it.data
// }
// }
//
// /**
// * 租户成员自己获取被授权的资源列表
// */
// fun tenantMemberGetResourceList(
// tenantId: String,
// resourceType:String): HttpCall, UserPoolAdminGetTenantAdminResourceList> {
// return client.createHttpGetCall(
// "${client.host}/api/v2/users/me/tenant/authorized-resources?tenant_id=${tenantId}&resource_type=${resourceType}",
// object : TypeToken>() {}) {
// it.data
// }
// }
//
// /**
// * 用户池管理员获取租户成员被授权的资源
// */
// fun userPoolAdminGetTenantMemberResourceList(
// tenantId: String,
// userId:String,
// resourceType:String): HttpCall, UserPoolAdminGetTenantAdminResourceList> {
// return client.createHttpGetCall(
// "${client.host}/api/v2/users/${userId}/tenant/authorized-resources?tenant_id=${tenantId}&user_id=${userId}&resource_type=${resourceType}",
// object : TypeToken>() {}) {
// it.data
// }
// }
/**
* 授权业务资源
*/
fun authorizeResources(options: AuthorizeResourcesParam): HttpCall {
return client.createHttpPostCall(
"${client.host}/api/v2/acl/authorize-resources",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
/**
* 撤销业务资源
*/
fun revokeAuthorizeResources(options: AuthorizeResourcesParam): HttpCall {
return client.createHttpPostCall(
"${client.host}/api/v2/acl/revoke-resources",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
/**
* 获取被授权的资源
*/
fun getAuthorizeResources(options: GetAuthorizeResourcesParam): HttpCall, RestfulResponse> {
return client.createHttpPostCall(
"${client.host}/api/v2/acl/list-authorized-resources",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it
}
}
/**
* 获取被授权的资源(用户侧)
*/
fun getMeAuthorizeResources(
namespace: String,
tenant_id: String,
resource_type: String
): HttpCall, UserPoolAdminGetTenantAdminResourceList> {
return client.createHttpGetCall(
"${client.host}/api/v2/acl/users/me/authorized-resources?namespace=${namespace}&tenant_id=${tenant_id}&resource_type=${resource_type}",
object : TypeToken>() {}) {
it.data
}
}
/**
* 批量获取被授权的资源
*/
fun getAuthorizeResourcesBatch(options: BatchGetAuthorizeResourcesParam): HttpCall, RestfulResponse> {
return client.createHttpPostCall(
"${client.host}/api/v2/acl/list-authorized-resources-batch",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it
}
}
/**
* 判断用户是否能操作某个资源
*/
fun isAllowed(
options: IsAllowedParam
): HttpCall, RestfulResponse> {
return client.createHttpPostCall(
"${client.host}/api/v2/acl/is-allowed",
GsonBuilder().create().toJson(options),
object : TypeToken>() {}
) {
it
}
}
/**
* 批量新增资源
*/
fun batchInsertResource(options: BatchResourceParam): HttpCall {
return client.createHttpPostCall(
"${client.host}/api/v2/resources/bulk",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy