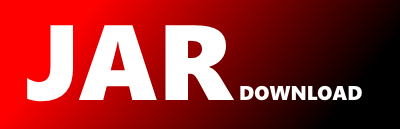
cn.authing.core.mgmt.UsersManagementClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-core Show documentation
Show all versions of java-core Show documentation
java backend sdk for authing
package cn.authing.core.mgmt
import cn.authing.core.Utils
import cn.authing.core.graphql.GraphQLCall
import cn.authing.core.graphql.GraphQLResponse
import cn.authing.core.http.HttpCall
import cn.authing.core.types.*
import com.google.gson.Gson
import com.google.gson.GsonBuilder
import com.google.gson.reflect.TypeToken
import java.util.*
/**
* 用户管理类
*/
class UsersManagementClient(private val client: ManagementClient) {
/**
* 获取用户列表
*/
@JvmOverloads
fun list(
page: Int? = null,
limit: Int? = null,
sortBy: SortByEnum? = null
): GraphQLCall {
val param = UsersParam(page, limit, sortBy)
return list(param)
}
/**
* 获取用户列表
*/
fun list(param: UsersParam): GraphQLCall {
if (null == param.withCustomData || false == param.withCustomData) {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
var param1 = UsersWithCustomDataParam(param.page,param.limit,param.sortBy);
return client.createGraphQLCall(
param1.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 创建用户
*/
@JvmOverloads
fun create(userInfo: CreateUserInput, options: CreateUserOptions? = null): HttpCall, User> {
val param = CreateUserParam(userInfo).withKeepPassword(options?.keepPassword).withResetPasswordOnFirstLogin(options?.resetPasswordOnFirstLogin)
if (param.userInfo.password !== null) {
param.userInfo.password = client.encrypt(param.userInfo.password!!)
}
return client.createHttpPostCall(
"${client.host}/api/v2/users",
GsonBuilder().create().toJson(param),
object : TypeToken> () {}
) { it.data }
}
/**
* 修改用户资料
*/
fun update(userId: String, updates: UpdateUserInput): HttpCall, User> {
if (updates.password != null && !updates.password.equals("")) {
updates.password = client.encrypt(updates.password)
}
return client.createHttpPostCall(
"${client.host}/api/v2/users/${userId}",
GsonBuilder().create().toJson(updates),
object : TypeToken> () {}
) {
it.data
}
}
/**
* 获取用户详情
*/
fun detail(userId: String, withCustomData: Boolean,withIdentities:Boolean): HttpCall, User> {
return client.createHttpGetCall(
"${client.host}/api/v2/users/$userId?with_custom_data=${withCustomData}&with_identities=${withIdentities}",
object : TypeToken> () {}
) { it.data }
}
/**
* 搜索用户
*/
@JvmOverloads
fun search(
query: String, fields: List? = null, page: Int? = null, limit: Int? = null
): GraphQLCall {
val param = SearchUserParam(query, fields, page, limit)
return search(param)
}
/**
* 搜索用户
*/
fun search(param: SearchUserParam): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 查找用户
*/
fun find(param: FindUserParam): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 通过 ID、username、email、phone、email、externalId 批量获取用户详情
*/
@JvmOverloads
fun batch(
identifiers: List,
options: BatchGetUserOptions? = null
): HttpCall>, List> {
return client.createHttpPostCall(
"${client.host}/api/v2/users/batch",
Gson().toJson(BatchGetUserPostData(identifiers, options?.queryField)),
object : TypeToken>>() {}) {
it.data
}
}
/**
* 删除用户
*/
fun delete(userId: String): GraphQLCall {
val param = DeleteUserParam(userId)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 批量删除用户
*/
fun deleteMany(userIds: List): GraphQLCall {
val param = DeleteUsersParam(userIds)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 检查用户是否存在,目前可检测的字段有用户名、邮箱、手机号。
*/
fun exists(param: IsUserExistsParam): HttpCall, Boolean> {
val url = "${client.host}/api/v2/users/is-user-exists"
return client.createHttpGetCall(
Utils().getQueryUrl(url, param),
object : TypeToken> () {}
) {
it.data
}
}
/**
* 获取用户角色列表
* TODO: 高版本删除
*/
@Deprecated("请使用listRoles(userId: String, namespace: String?)替换此方法")
fun listRoles(userId: String): HttpCall, PaginatedRoles> {
return listRoles(userId, null);
}
/**
* 获取用户角色列表
*/
fun listRoles(userId: String, namespace: String?): HttpCall, PaginatedRoles> {
var url = "${client.host}/api/v2/users/${userId}/roles"
url += if (namespace != null) "?namespace=${namespace}" else ""
return client.createHttpGetCall(
url,
object : TypeToken> () {}
) {
it.data
}
}
/**
* 将用户加入角色
* TODO: 高版本删除
*/
@Deprecated("请使用addRoles(userId: String, roles: List, namespace: String?)替换此方法")
fun addRoles(userId: String, roleCodes: List): HttpCall, CommonMessage> {
return addRoles(userId, roleCodes, null);
}
/**
* 将用户加入角色
*/
fun addRoles(
userId: String,
roleCodes: List,
namespace: String?
): HttpCall, CommonMessage> {
val options = RestAddRolesParams(userId = userId, namespace = namespace, list = roleCodes)
return client.createHttpPostCall(
"${client.host}/api/v2/users/${options.userId}/roles",
GsonBuilder().create().toJson(options),
object : TypeToken> () {}
) {
it.data
}
}
/**
* 将用户从角色中移除
* TODO: 高版本删除
*/
@Deprecated("请使用removeRoles(userId: String, roles: List, namespace: String?)替换此方法")
fun removeRoles(userId: String, roleCodes: List): GraphQLCall {
return removeRoles(userId, roleCodes, null);
}
/**
* 将用户从角色中移除
*/
fun removeRoles(
userId: String,
roleCodes: List,
namespace: String?
): GraphQLCall {
val param = RevokeRoleParam().withUserIds(listOf(userId)).withRoleCodes(roleCodes).withNamespace(namespace)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 刷新用户 token
*/
fun refreshToken(userId: String): GraphQLCall {
val param = RefreshTokenParam(userId)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 获取用户分组列表
*/
fun listGroups(userId: String): GraphQLCall {
val param = GetUserGroupsParam(userId)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result.groups!!
}
}
/**
* 将用户加入分组
*/
fun addGroup(userId: String, group: String): GraphQLCall {
val param = AddUserToGroupParam(listOf(userId), group)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 将用户退出分组
*/
fun removeGroup(userId: String, group: String): GraphQLCall {
val param = RemoveUserFromGroupParam(listOf(userId), group)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 获取策略列表
*/
@JvmOverloads
fun listPolicies(
userId: String,
page: Int = 1,
limit: Int = 10
): GraphQLCall {
val param =
PolicyAssignmentsParam().withTargetType(PolicyAssignmentTargetType.USER).withTargetIdentifier(userId)
.withPage(page).withLimit(limit)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 批量添加策略
*/
fun addPolicies(
userId: String,
policies: List
): GraphQLCall {
val param =
AddPolicyAssignmentsParam(policies, PolicyAssignmentTargetType.USER).withTargetIdentifiers(listOf(userId))
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 批量移除策略
*/
fun removePolicies(
userId: String,
policies: List
): GraphQLCall {
val param = RemovePolicyAssignmentsParam(
policies,
PolicyAssignmentTargetType.USER
).withTargetIdentifiers(listOf(userId))
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 获取当前用户的自定义数据列表
*/
fun listUdv(userId: String): GraphQLCall> {
val param = UdvParam(UdfTargetType.USER, userId)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 添加自定义数据
*/
fun setUdv(userId: String, key: String, value: Any): GraphQLCall> {
val json = Gson()
val param = SetUdvParam(UdfTargetType.USER, userId, key, json.toJson(value))
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 移除自定义数据
*/
fun removeUdv(userId: String, key: String): GraphQLCall> {
val param = RemoveUdvParam(UdfTargetType.USER, userId, key)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 获取用户所在组织机构
*/
fun listOrgs(userId: String): HttpCall>>, List>> {
return client.createHttpGetCall(
"${client.host}/api/v2/users/${userId}/orgs",
object : TypeToken>>>() {}) {
it.data
}
}
/**
* 获取用户被授权的所有资源
*/
fun listAuthorizedResources(
userId: String,
namespace: String
): GraphQLCall {
val param = ListUserAuthorizedResourcesParam(userId, namespace)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result.authorizedResources!!
}
}
/**
* 获取用户被授权的所有资源
*/
fun listAuthorizedResources(
param: ListUserAuthorizedResourcesParam
): GraphQLCall {
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result.authorizedResources!!
}
}
/**
* 获取某个用户的所有自定义数据
*
*/
fun getUdfValue(userId: String): HttpCall>, Map> {
return client.createHttpGetCall(
"${client.host}/api/v2/udfs/values?targetId=${userId}&targetType=${UdfTargetType.USER}",
object : TypeToken>> () {}
) {
convertUdvToKeyValuePair(it.data)
}
}
/**
* 批量获取多个用户的自定义数据
*
*/
fun getUdfValueBatch(userIds: List): GraphQLCall>> {
if (userIds.isEmpty()) {
throw Exception("userIds can't be null")
}
val param = UdfValueBatchParam(UdfTargetType.USER, userIds)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
val hashtable = Hashtable>()
it.result.map { hashtable.put(it.targetId, convertUdvToKeyValuePair(it.data)) }
hashtable
}
}
/**
* 设置某个用户的自定义数据
*/
fun setUdfValue(
userId: String,
data: Map
): HttpCall>, List> {
val params = RestSetUdfValueParams(UdfTargetType.USER, userId, data)
return client.createHttpPostCall(
"${client.host}/api/v2/udvs",
GsonBuilder().create().toJson(params),
object : TypeToken>> () {}
) {
it.data
}
}
/**
* 批量设置自定义数据
*/
fun setUdfValueBatch(input: List): GraphQLCall> {
if (input.isEmpty()) {
throw Exception("empty input list")
}
val inputList = input.flatMap { item ->
item.data.map { SetUdfValueBatchInput(item.userId, it.key, Gson().toJson(it.value)) }
}
println(input)
val param = SetUdfValueBatchParam(UdfTargetType.USER, inputList)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {})
{
it.result
}
}
/**
* 清除用户的自定义数据
*/
fun removeUdfValue(userId: String, key: String): GraphQLCall> {
return removeUdv(userId, key);
}
/**
* 判断用户是否有某个角色
*/
fun hasRole(
option: IHasRoleParam
): Boolean {
val (totalCount, list) = this.listRoles(option.userId, option.namespace).execute()
if (totalCount < 1) return false
var hasRole = false;
list.forEach { item -> if (item.code == option.roleCode) hasRole = true }
return hasRole
}
/**
* 强制一批用户下线
*/
fun kick(
userIds: List
): HttpCall, Boolean> {
val url =
"${client.host}/api/v2/users/kick"
val body = "{ \"userIds\": ${GsonBuilder().create().toJson(userIds)} }"
return this.client.createHttpPostCall(
url,
body,
object : TypeToken>() {}) { it.code == 200 }
}
/**
* 查看用户操作日志
*/
@JvmOverloads
fun listUserActions(
options: ListUserActionsParams? = ListUserActionsParams()
): HttpCall, UserActions> {
var url = "${client.host}/api/v2/analysis/user-action?page=${options?.page}&limit=${options?.limit}"
url += if (options?.clientIp != null) "&clientip=${options.clientIp}" else ""
url += if (options?.operationName != null) "&operation_name=${options.operationName}" else ""
url += if (options?.operatoArn != null) "&operator_arn=${options.operatoArn}" else ""
return client.createHttpGetCall(
url,
object : TypeToken>() {}
) { it.data }
}
/**
* 获取用户所在部门
*/
fun listDepartment(
userId: String
): HttpCall>, Pagination> {
return client.createHttpGetCall(
"${client.host}/api/v2/users/${userId}/departments",
object : TypeToken>> () {}
) {
it.data
}
}
/**
* 检查用户登录状态
*/
fun checkLoginStatus(
param: CheckLoginStatusParams
): HttpCall, UserCheckLoginStatusResponse> {
var url = "${client.host}/api/v2/users/login-status?userId=${param.userId}"
url += if (param.appId != null) "&appId=${param.appId}" else ""
url += if (param.deviceId != null) "&deviceId=${param.deviceId}" else ""
return client.createHttpGetCall(
url,
object : TypeToken>() {}) { it.data }
}
/**
* 用户池管理员手动将任意一个身份绑定到一个用户上
*/
fun identityLink(options:IdentityLinkParam):HttpCall {
return client.createHttpPostCall(
"${client.host}/api/v2/users/identity/link",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
/**
*用户池管理员手动给用户解绑身份信息,例如解绑用户 A 的微信身份
*/
fun unIdentityLink(options:UnIdentityLinkParam):HttpCall {
return client.createHttpPostCall(
"${client.host}/api/v2/users/identity/unlink",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
/**
*
解除用户某个身份源下的所有身份(以用户身份调用)
*/
fun unlinkByUser(options:UnlinkByUserParam):HttpCall {
return client.createHttpPostCall(
"${client.host}/api/v2/users/identity/unlinkByUser",
GsonBuilder().create().toJson(options),
object : TypeToken() {}
) {
it
}
}
/**
* 用户退出登录
*/
fun logout(options: UserLogoutParams): HttpCall, Boolean> {
var url = "${client.host}/logout?userId=${options.userId}"
url += if (options.appId != null) "&appId=${options.appId}" else ""
return client.createHttpGetCall(
url,
object : TypeToken> () {}
) {
it.code == 200
}
}
/**
* 获取已归档用户列表
*/
fun listArchivedUsers(page: Int, limit: Int): GraphQLCall {
val param = ArchivedUsersParam(page, limit)
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.result
}
}
/**
* 发送首次登录验证邮件
*/
fun sendFirstLoginVerifyEmail(appId: String, userId: String): GraphQLCall {
val param = SendFirstLoginVerifyEmailParam(appId, userId);
return client.createGraphQLCall(
param.createRequest(),
object : TypeToken>() {}) {
it.sendFirstLoginVerifyEmail
}
}
/**
* 获取用户所在租户
*/
fun getUserTenants(userId: String): HttpCall, UserTenantResponse> {
val url = "${client.host}/api/v2/users/${userId}/tenants"
return client.createHttpGetCall(
url,
object : TypeToken> () {}
) {
it.data
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy