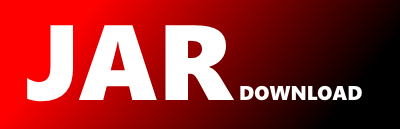
cn.baiweigang.qtaf.ift.testcase.autocreate.IftDataFileCase Maven / Gradle / Ivy
package cn.baiweigang.qtaf.ift.testcase.autocreate;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
import cn.baiweigang.qtaf.dispatch.testcase.SuperCase;
import cn.baiweigang.qtaf.ift.IftConf;
import cn.baiweigang.qtaf.toolkit.util.CommUtils;
import cn.baiweigang.qtaf.toolkit.util.LogUtil;
import cn.baiweigang.qtaf.toolkit.util.StringUtil;
/**
* 数据文件类型的测试用例
* @author @bwgang
*
*/
public class IftDataFileCase extends SuperCase{
private LogUtil log =LogUtil.getLogger(IftDataFileCase.class);//日志记录
//任务名称
private String taskName;//测试任务名称
//根据用例数据文件,创建java、xml文件相关配置信息
private String javaCase;//生成的java文件存储目录
private String times;// 获取当前时间戳
private String allReportPath ;// html、excel测试报告存储的上级目录
private String htmlReportPath ;// excel格式测试报告存储的目录
private String excelReportPath ;// excel格式测试报告存储的目录
private String reportExcelName ;// excel格式测试报告名称
private String xmlFilePath ;// 生成xml文件的路径
private String xmlFileName ;// 生成xml文件的名称
private String packageInfo;//生成java文件的包名
private TestngXmlSuite xmlSuite;//用例自动生成用到的测试套
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy