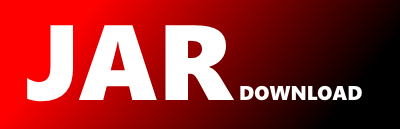
cn.bestwu.api.test.ApiTestConfiguration Maven / Gradle / Ivy
package cn.bestwu.api.test;
import cn.bestwu.api.sign.ApiSignProperties;
import com.github.stuxuhai.jpinyin.PinyinHelper;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import javax.annotation.PostConstruct;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.boot.autoconfigure.condition.ConditionalOnWebApplication;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.util.Assert;
import org.springframework.util.DigestUtils;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.ViewControllerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
/**
* 系统安全配置
*
* @author Peter Wu
*/
@Configuration
@ConditionalOnWebApplication
@EnableConfigurationProperties(ApiProperties.class)
public class ApiTestConfiguration {
@ConditionalOnMissingBean(ApiTestUserRepository.class)
@Bean
@ConditionalOnWebApplication
public ApiTestUserRepository apiTestUserRepository(final ApiProperties properties) {
return new ApiTestUserRepository() {
@Override
public ApiTestUser findByUsername(String username) {
return new ApiTestUser() {
@Override
public String getUsername() {
return properties.getUsername();
}
@Override
public String getPassword() {
return DigestUtils
.md5DigestAsHex((getUsername() + properties.getPassword()).getBytes());
}
};
}
};
}
@Bean
public RememberMeService rememberMeService(ApiTestUserRepository apiTestUserRepository,
ApiProperties properties) {
return new RememberMeService(properties.getRememberMeKey(), apiTestUserRepository);
}
@Configuration
@ConditionalOnWebApplication
protected static class WebMvcConfigurer extends WebMvcConfigurerAdapter {
@Autowired
private ApiProperties properties;
private String testPath;
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler(testPath + "/**")
.addResourceLocations(properties.getResourceLocations())
.setCachePeriod(60 * 10);
}
@Override
public void addViewControllers(ViewControllerRegistry registry) {
registry.addRedirectViewController(testPath, testPath + "/index.html");
registry.addRedirectViewController(testPath + "/", testPath + "/index.html");
}
private String[] apiPath;
@PostConstruct
public void init() {
testPath = properties.getPath();
apiPath = new String[]{testPath + "/path", testPath + "/pinyin", testPath + "/api.json",
testPath + "/code.json", testPath + "/datastructure.json", testPath + "/field.json",
testPath + "/tree.json",
testPath + "/doc/**"};
}
@Bean
@ConditionalOnProperty(prefix = "api.test", name = "dev", havingValue = "false", matchIfMissing = true)
public FilterRegistrationBean apiTestFilter(RememberMeService rememberMeService) {
FilterRegistrationBean registrationBean = new FilterRegistrationBean(
new ApiTestFilter(rememberMeService));
registrationBean.addUrlPatterns(apiPath);
registrationBean.setOrder(FilterRegistrationBean.REQUEST_WRAPPER_FILTER_MAX_ORDER - 9800);
return registrationBean;
}
}
@ConditionalOnWebApplication
@RestController
@RequestMapping(name = "测试", value = "${api.test.path:/_t}")
protected static class ApiTestController {
private Logger log = LoggerFactory.getLogger(ApiTestController.class);
private String defaultApiTestSigninUserKey = ApiTestFilter.DEFAULT_API_TEST_SIGNIN_USER_KEY;
private final RememberMeService rememberMeService;
private final ApiTestUserRepository apiTestUserRepository;
private final ApiProperties properties;
@Autowired
public ApiTestController(RememberMeService rememberMeService,
ApiTestUserRepository apiTestUserRepository, ApiProperties properties) {
this.rememberMeService = rememberMeService;
this.apiTestUserRepository = apiTestUserRepository;
this.properties = properties;
}
@RequestMapping(name = "登录", value = "/login", method = RequestMethod.POST)
public Object login(String username, String password,
@RequestParam(defaultValue = "false") Boolean remeberMe,
HttpServletRequest request, HttpServletResponse response) {
Assert.hasText(username, "请输入用户名");
Assert.hasText(password, "请输入密码");
ApiTestUser apiTestUser = apiTestUserRepository.findByUsername(username);
if (apiTestUser == null || !password.equals(apiTestUser.getPassword())) {
return ResponseEntity.status(HttpStatus.UNPROCESSABLE_ENTITY)
.body(Collections.singletonMap("message", "用户名或密码不正确"));
}
request.getSession().setAttribute(defaultApiTestSigninUserKey, apiTestUser);
if (remeberMe) {
rememberMeService.onLoginSuccess(request, response, username, password);
}
return ResponseEntity.noContent().build();
}
@Autowired(required = false)
private ApiSignProperties apiSignProperties;
/**
* @return 公共属性
*/
@RequestMapping(name = "公共属性", value = "/properties", method = RequestMethod.GET)
public Object path() {
Map map = new HashMap<>();
map.put("path", properties.getPath());
map.put("devDoc", properties.getDevDoc());
if (apiSignProperties != null) {
map.put("client_id", apiSignProperties.getClient_id());
map.put("client_secret", apiSignProperties.getClient_secret());
map.put("mode", apiSignProperties.getMode().ordinal());
}
String etag = "\"".concat(Sha1DigestUtil.shaHex(map.toString().getBytes())).concat("\"");
return ResponseEntity.ok().header(HttpHeaders.ETAG, etag).body(map);
}
/**
* 拼音
*
* @param word word
* @return word对应简拼
*/
@RequestMapping(name = "拼音", value = "/pinyin", method = RequestMethod.GET)
public Object pinyin(String[] word) {
Assert.notEmpty(word, "word不能为空");
Map map = new HashMap<>();
for (String w : word) {
try {
map.put(w, PinyinHelper.getShortPinyin(w));
} catch (Exception e) {
log.error(e.getMessage(), e);
}
}
String etag = "\"".concat(Sha1DigestUtil.shaHex(map.toString().getBytes())).concat("\"");
return ResponseEntity.ok().header(HttpHeaders.ETAG, etag).body(map);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy