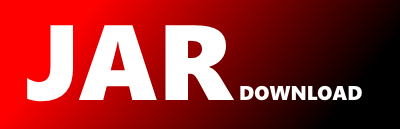
cn.bestwu.umeng.push.PushClient Maven / Gradle / Ivy
package cn.bestwu.umeng.push;
import cn.bestwu.umeng.push.android.AndroidNotification;
import cn.bestwu.umeng.push.ios.IOSNotification;
import org.json.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.util.DigestUtils;
import org.springframework.web.client.RestTemplate;
import java.time.Instant;
/**
* 推送客户端
*
* @author Peter Wu
*/
public class PushClient {
protected Logger logger = LoggerFactory.getLogger(this.getClass());
private NotificationProperties android;
private NotificationProperties ios;
protected final RestTemplate restTemplate;
// The host
protected final String host = "http://msg.umeng.com";
// The upload path
protected final String uploadPath = "/upload";
// The post path
protected final String postPath = "/api/send";
public PushClient(PushProperties properties, RestTemplate restTemplate) {
this.restTemplate = restTemplate;
android = properties.getAndroid();
if (android == null) {
android = properties;
}
ios = properties.getIos();
if (ios == null) {
ios = properties;
}
}
private JSONObject readValue(String result) {
return new JSONObject(result);
}
/**
* 推送消息
*
* @param notification 信息
*/
private void sendNotification(UmengNotification notification) {
String timestamp = String.valueOf(Instant.now().getEpochSecond());
notification.setPredefinedKeyValue("timestamp", timestamp);
String url = host + postPath;
String postBody = notification.getPostBody();
String sign = DigestUtils.md5DigestAsHex(("POST" + url + postBody + notification.getAppMasterSecret()).getBytes());
url = url + "?sign=" + sign;
ResponseEntity entity = restTemplate.postForEntity(url, postBody, String.class);
if (entity.getStatusCode().equals(HttpStatus.OK)) {
if (logger.isDebugEnabled())
logger.debug("Notification sent successfully.\nresult: " + entity.getBody());
} else {
throw new PushException(readValue(entity.getBody()).getJSONObject("data").getString("error_code"), entity.getBody());
}
}
/**
* Upload file with device_tokens to Umeng
*
* @param appkey appkey
* @param appMasterSecret appMasterSecret
* @param contents device tokens use '\n' to split them if there are multiple tokens
* @return 返回 file_id
*/
private String uploadContents(String appkey, String appMasterSecret, String contents) {
// Construct the json string
JSONObject uploadJson = new JSONObject();
uploadJson.put("appkey", appkey);
String timestamp = String.valueOf(Instant.now().getEpochSecond());
uploadJson.put("timestamp", timestamp);
uploadJson.put("content", contents);
// Construct the request
String url = host + uploadPath;
String postBody = uploadJson.toString();
String sign = DigestUtils.md5DigestAsHex(("POST" + url + postBody + appMasterSecret).getBytes());
url = url + "?sign=" + sign;
ResponseEntity entity = restTemplate.postForEntity(url, postBody, String.class);
JSONObject body = readValue(entity.getBody());
String ret = body.getString("ret");
if (!ret.equals("SUCCESS")) {
throw new PushException(body.getJSONObject("data").getString("error_code"), entity.getBody());
}
JSONObject data = body.getJSONObject("data");
return data.getString("file_id");
}
/**
* 推送安卓消息
*
* @param notification 信息
*/
public void send(AndroidNotification notification) {
notification.setAppMasterSecret(android.getAppMasterSecret());
notification.setPredefinedKeyValue("appkey", android.getAppkey());
notification.setPredefinedKeyValue("production_mode", android.isProductionMode());
sendNotification(notification);
}
/**
* 推送安卓消息
*
* @param notification 信息
*/
public void send(IOSNotification notification) {
notification.setAppMasterSecret(ios.getAppMasterSecret());
notification.setPredefinedKeyValue("appkey", ios.getAppkey());
notification.setPredefinedKeyValue("production_mode", ios.isProductionMode());
sendNotification(notification);
}
/**
* Upload file with IOS device_tokens to Umeng
*
* @param contents contents device tokens use '\n' to split them if there are multiple tokens
* @return 返回 file_id
*/
public String uploadIOSContents(String contents) {
return uploadContents(ios.getAppkey(), ios.getAppMasterSecret(), contents);
}
/**
* Upload file with Android device_tokens to Umeng
*
* @param contents contents device tokens use '\n' to split them if there are multiple tokens
* @return 返回 file_id
*/
public String uploadAndroidContents(String contents) {
return uploadContents(android.getAppkey(), android.getAppMasterSecret(), contents);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy