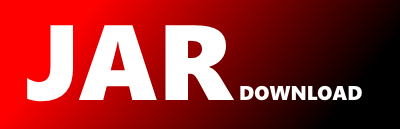
cn.blankcat.demo.HelloWordMessageHandler Maven / Gradle / Ivy
package cn.blankcat.demo;
import cn.blankcat.dto.message.Message;
import cn.blankcat.dto.message.MessageToCreate;
import cn.blankcat.openapi.MessageService;
import cn.blankcat.openapi.OpenapiService;
import cn.blankcat.websocket.WebsocketService;
import cn.blankcat.websocket.handler.WebsocketHandler;
import java.io.IOException;
public class HelloWordMessageHandler implements WebsocketHandler {
@Override
public void handle(String message) {
Message guildMessage = toType(message, Message.class);
if (guildMessage.getContent().contains("@")){
OpenapiService openapiService = new OpenapiService();
MessageToCreate messageToCreate = new MessageToCreate();
messageToCreate.setContent("hello world");
messageToCreate.setMsgID(guildMessage.getId());
try {
openapiService.create(MessageService.class).postChannelMessages(guildMessage.getChannelId(), messageToCreate).execute().body();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
WebsocketService websocketService = new WebsocketService();
new HelloWordMessageHandler().register(Message.class);
websocketService.connect();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy