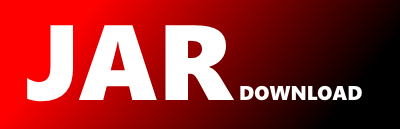
cn.bootx.table.modify.mysql.service.MySqlCreateTableService Maven / Gradle / Ivy
package cn.bootx.table.modify.mysql.service;
import cn.bootx.table.modify.constants.TableCharset;
import cn.bootx.table.modify.mysql.constants.MySqlEngineEnum;
import cn.bootx.table.modify.mysql.entity.*;
import cn.bootx.table.modify.mysql.handler.MySqlTableModifyDao;
import cn.bootx.table.modify.mysql.util.MySqlInfoUtil;
import cn.bootx.table.modify.mysql.util.MySqlTableModifyUtil;
import cn.bootx.table.modify.properties.TableModifyProperties;
import cn.hutool.core.collection.CollUtil;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
/**
* MySQL表信息创建处理
* @author xxm
* @since 2023/4/19
*/
@Slf4j
@Service
@RequiredArgsConstructor
public class MySqlCreateTableService {
private final MySqlTableModifyDao mySqlTableModifyDao;
private final TableModifyProperties tableModifyProperties;
/**
* 根据map结构创建表
* @param modifyMap 用于存需要创建表的数据
*/
public void createTable(MySqlModifyMap modifyMap) {
// 做创建表操作
for (MySqlEntityTable table : modifyMap.getCreateTables()) {
log.info("开始创建表:" + table.getName());
try {
MySqlCreateParam createParam = this.buildCreateParam(table, modifyMap);
// 表字段为空不进行创建
if (CollUtil.isEmpty(createParam.getColumns())){
log.warn("扫描发现 {} 没有建表字段,请检查!",createParam.getName());
}
String createSql = this.buildCreateSql(createParam);
mySqlTableModifyDao.createTable(createSql);
log.info("完成创建表:" + table.getName());
} catch (Exception e){
log.error("创建表失败:" + table.getName(),e);
// 快速失败
if (tableModifyProperties.isFailFast()){
throw e;
}
}
}
}
/**
* 构建建表条件
*/
private MySqlCreateParam buildCreateParam(MySqlEntityTable table, MySqlModifyMap modifyMap){
MySqlCreateParam mySqlCreateParam = new MySqlCreateParam();
// 表基础信息
mySqlCreateParam.setName(table.getName())
.setEngine(Optional.ofNullable(table.getEngine()).map(MySqlEngineEnum::getValue).orElse(null))
.setComment(table.getComment())
.setCharset(Optional.ofNullable(table.getCharset()).map(TableCharset::getValue).orElse(null));
// 字段
List columns = modifyMap.getAddColumns()
.get(table.getName());
if (CollUtil.isNotEmpty(columns)) {
List list = columns.stream()
.map(MySqlEntityColumn::toColumn)
.collect(Collectors.toList());
mySqlCreateParam.setColumns(list);
}
// 主键
List keys = table.getKeys();
if (CollUtil.isNotEmpty(keys)){
mySqlCreateParam.setKeys(MySqlInfoUtil.buildBracketParams(keys));
}
// 索引
List indexes = modifyMap.getAddIndexes()
.get(table.getName());
if (CollUtil.isNotEmpty(indexes)){
List list = indexes.stream()
.map(MySqlEntityIndex::toIndex)
.collect(Collectors.toList());
mySqlCreateParam.setIndexes(list);
}
return mySqlCreateParam;
}
/**
* 构造查询语句
*/
private String buildCreateSql(MySqlCreateParam param){
StringBuilder sql = new StringBuilder();
// 表名
sql.append(MySqlTableModifyUtil.tableName(param));
sql.append("(");
// 列
sql.append(MySqlTableModifyUtil.column(param));
// 索引
sql.append(MySqlTableModifyUtil.primaryKey(param));
sql.append(MySqlTableModifyUtil.index(param));
// 去除最后的逗号
sql.deleteCharAt(sql.length()-1);
sql.append(")");
// 引擎类型
sql.append(MySqlTableModifyUtil.engine(param));
// 字符集
sql.append(MySqlTableModifyUtil.charset(param));
// 备注
sql.append(MySqlTableModifyUtil.comment(param));
return sql.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy