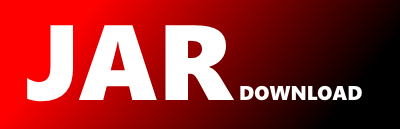
cn.bootx.table.modify.mysql.service.MySqlModifyTableService Maven / Gradle / Ivy
package cn.bootx.table.modify.mysql.service;
import cn.bootx.table.modify.constants.UpdateType;
import cn.bootx.table.modify.mysql.entity.*;
import cn.bootx.table.modify.mysql.handler.MySqlTableModifyDao;
import cn.bootx.table.modify.mysql.util.MySqlInfoUtil;
import cn.bootx.table.modify.mysql.util.MySqlTableModifyUtil;
import cn.bootx.table.modify.properties.TableModifyProperties;
import cn.hutool.core.collection.CollUtil;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
/**
* MySQL表信息修改处理
* @author xxm
* @since 2023/4/7
*/
@Slf4j
@Service
@RequiredArgsConstructor
public class MySqlModifyTableService {
private final MySqlTableModifyDao mySqlTableModifyDao;
private final TableModifyProperties tableModifyProperties;
/**
* 根据传入的map创建或修改表结构
* @param baseTableMap 操作sql的数据结构
*/
public void modifyTableConstruct(MySqlModifyMap baseTableMap) {
List updateTables = baseTableMap.getUpdateTables();
for (MySqlTableUpdate table : updateTables) {
MySqlModifyParam mySqlModifyParam = this.buildModifyParam(table, baseTableMap);
if (mySqlModifyParam.isUpdate()) {
log.info("开始更新表:" + table.getName());
try {
String modifyTableSql = this.buildModifySql(mySqlModifyParam);
mySqlTableModifyDao.modifyTable(modifyTableSql);
log.info("完成更新表:" + table.getName());
} catch (Exception e){
log.error("更新表失败:" + table.getName(),e);
// 快速失败
if (tableModifyProperties.isFailFast()){
throw e;
}
}
}
}
}
/**
* 构建建表语句
*/
private MySqlModifyParam buildModifyParam(MySqlTableUpdate table, MySqlModifyMap modifyMap){
MySqlModifyParam mySqlModifyParam = new MySqlModifyParam();
// 表基础信息
mySqlModifyParam.setName(table.getName())
.setEngine(table.getEngine())
.setEngineUpdate(table.isEngineUpdate())
.setComment(table.getComment())
.setCommentUpdate(table.isCommentUpdate())
.setCharset(table.getCharset())
.setCharsetUpdate(table.isCharsetUpdate());
// 主键是否更新
if (table.isKeysUpdate()){
mySqlModifyParam.setKeys(MySqlInfoUtil.buildBracketParams(table.getKeys()));
}
UpdateType updateType = tableModifyProperties.getUpdateType();
// 新增字段处理
if (CollUtil.isNotEmpty(modifyMap.getAddColumns().get(table.getName()))) {
List columns = modifyMap.getAddColumns()
.get(table.getName())
.stream()
.map(MySqlEntityColumn::toColumn)
.collect(Collectors.toList());
mySqlModifyParam.setAddColumns(columns);
}
// 更新字段
if (updateType != UpdateType.CREATE){
if (CollUtil.isNotEmpty(modifyMap.getUpdateColumns().get(table.getName()))) {
List columns = modifyMap.getUpdateColumns()
.get(table.getName())
.stream()
.map(MySqlEntityColumn::toColumn)
.collect(Collectors.toList());
mySqlModifyParam.setModifyColumns(columns);
}
}
// 删除字段
if (updateType != UpdateType.CREATE){
if (CollUtil.isNotEmpty(modifyMap.getDropColumns().get(table.getName()))) {
mySqlModifyParam.setDropColumns(modifyMap.getDropColumns().get(table.getName()));
}
}
// 删除索引
if (updateType != UpdateType.CREATE){
List delIndexes = new ArrayList<>();
// 要进行删除的索引
if (CollUtil.isNotEmpty(modifyMap.getDropIndexes().get(table.getName()))) {
delIndexes.addAll(modifyMap.getDropIndexes().get(table.getName()));
}
// 更新的索引需要先删后增
if (CollUtil.isNotEmpty(modifyMap.getUpdateIndexes().get(table.getName()))){
List list = modifyMap.getUpdateIndexes().get(table.getName())
.stream()
.map(MySqlEntityIndex::getName)
.collect(Collectors.toList());
delIndexes.addAll(list);
}
if (CollUtil.isNotEmpty(delIndexes)){
mySqlModifyParam.setDropIndexes(delIndexes);
}
}
// 新增索引
if (CollUtil.isNotEmpty(modifyMap.getAddIndexes().get(table.getName()))) {
// 要进行添加的索引
List addIndexes = modifyMap.getAddIndexes().get(table.getName())
.stream()
.map(MySqlEntityIndex::toIndex)
.collect(Collectors.toList());
// 更新的索引需要先删后增
if (CollUtil.isNotEmpty(modifyMap.getUpdateIndexes().get(table.getName()))){{
List list = modifyMap.getUpdateIndexes().get(table.getName())
.stream()
.map(MySqlEntityIndex::toIndex)
.collect(Collectors.toList());
addIndexes.addAll(list);
}}
mySqlModifyParam.setAddIndexes(addIndexes);
}
return mySqlModifyParam;
}
/**
* 组装表更新语句
*/
private String buildModifySql(MySqlModifyParam param){
StringBuilder sql = new StringBuilder();
// 表名
sql.append(MySqlTableModifyUtil.tableName(param));
// 删除列
sql.append(MySqlTableModifyUtil.dropColumn(param));
// 更新列
sql.append(MySqlTableModifyUtil.updateColumn(param));
// 添加列
sql.append(MySqlTableModifyUtil.addColumn(param));
// 更新主键
sql.append(MySqlTableModifyUtil.primaryKey(param));
// 删除索引
sql.append(MySqlTableModifyUtil.dropIndex(param));
// 添加索引
sql.append(MySqlTableModifyUtil.addIndex(param));
// 修改表引擎
sql.append(MySqlTableModifyUtil.engine(param));
sql.append(MySqlTableModifyUtil.charset(param));
// 修改注释
sql.append(MySqlTableModifyUtil.comment(param));
sql.deleteCharAt(sql.length()-1);
sql.append(";");
return sql.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy