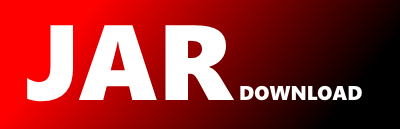
cn.tom.kit.StringUtil Maven / Gradle / Ivy
package cn.tom.kit;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.StringTokenizer;
public class StringUtil {
public static final String EMPTY = "";
public static final int INDEX_NOT_FOUND = -1;
public static boolean isEmpty(String str) {
return str == null || str.length() == 0;
}
public static boolean equals(String str1, String str2) {
return ((str1 == null) ? false : str1.equals(str2));
}
public static String escapeSql(String str) {
if (str == null) {
return null;
}
return str.replaceAll("'", "''");
}
public static String substringBefore(String str, String separator) {
if (isEmpty(str) || separator == null) {
return str;
}
if (separator.length() == 0) {
return EMPTY;
}
int pos = str.indexOf(separator);
if (pos == INDEX_NOT_FOUND) {
return str;
}
return str.substring(0, pos);
}
public static String substringBetween(String str, String tag) {
return substringBetween(str, tag, tag);
}
public static String substringBetween(String str, String open, String close) {
if (str == null || open == null || close == null) {
return null;
}
int start = str.indexOf(open);
if (start != INDEX_NOT_FOUND) {
int end = str.indexOf(close, start + open.length());
if (end != INDEX_NOT_FOUND) {
return str.substring(start + open.length(), end);
}
}
return null;
}
public static boolean contains(String str, String searchStr) {
if (str == null || searchStr == null) {
return false;
}
return str.indexOf(searchStr) >= 0;
}
public static String defaultIfEmpty(String str, String defaultStr) {
return isEmpty(str) ? defaultStr : str;
}
/**
* {null,"",null,2,3} --> 2,3
* {"",2,"",2,"",} --> 2,2
* @param obj
* @param split
* @return
*/
public static String join(Object[] obj, String split) {
StringBuffer str = new StringBuffer();
for(Object o: obj){
if(o==null || o.toString().isEmpty()) continue;
if(str.length()>0) str.append(split);
str.append(o);
}
return str.toString();
}
/**
* Tokenize the given String into a String array via a StringTokenizer.
* Trims tokens and omits empty tokens.
* The given delimiters string is supposed to consist of any number of
* delimiter characters. Each of those characters can be used to separate
* tokens. A delimiter is always a single character; for multi-character
* delimiters, consider using delimitedListToStringArray
* @param str the String to tokenize
* @param delimiters the delimiter characters, assembled as String
* (each of those characters is individually considered as delimiter).
* @return an array of the tokens
* @see java.util.StringTokenizer
* @see String#trim()
*/
public static String[] tokenizeToStringArray(String str, String delimiters) {
return tokenizeToStringArray(str, delimiters, true, true);
}
/**
* Tokenize the given String into a String array via a StringTokenizer.
*
The given delimiters string is supposed to consist of any number of
* delimiter characters. Each of those characters can be used to separate
* tokens. A delimiter is always a single character; for multi-character
* delimiters, consider using delimitedListToStringArray
* @param str the String to tokenize
* @param delimiters the delimiter characters, assembled as String
* (each of those characters is individually considered as delimiter)
* @param trimTokens trim the tokens via String's trim
* @param ignoreEmptyTokens omit empty tokens from the result array
* (only applies to tokens that are empty after trimming; StringTokenizer
* will not consider subsequent delimiters as token in the first place).
* @return an array of the tokens (null
if the input String
* was null
)
* @see java.util.StringTokenizer
* @see String#trim()
*/
public static String[] tokenizeToStringArray(
String str, String delimiters, boolean trimTokens, boolean ignoreEmptyTokens) {
if (str == null) {
return null;
}
StringTokenizer st = new StringTokenizer(str, delimiters);
List tokens = new ArrayList();
while (st.hasMoreTokens()) {
String token = st.nextToken();
if (trimTokens) {
token = token.trim();
}
if (!ignoreEmptyTokens || token.length() > 0) {
tokens.add(token);
}
}
return toStringArray(tokens);
}
/**
* Copy the given Collection into a String array.
* The Collection must contain String elements only.
* @param collection the Collection to copy
* @return the String array (null
if the passed-in
* Collection was null
)
*/
public static String[] toStringArray(Collection collection) {
if (collection == null) {
return null;
}
return collection.toArray(new String[collection.size()]);
}
/**
* Check whether the given String has actual text.
* More specifically, returns true
if the string not null
,
* its length is greater than 0, and it contains at least one non-whitespace character.
* @param str the String to check (may be null
)
* @return true
if the String is not null
, its length is
* greater than 0, and it does not contain whitespace only
* @see #hasText(CharSequence)
*/
public static boolean hasText(String str) {
return hasText((CharSequence) str);
}
public static boolean hasText(CharSequence str) {
if (!hasLength(str)) {
return false;
}
int strLen = str.length();
for (int i = 0; i < strLen; i++) {
if (!Character.isWhitespace(str.charAt(i))) {
return true;
}
}
return false;
}
/**
* 检查包含空白字符在内的字符系列长度
*
* StringUtils.hasLength(null) = false
* StringUtils.hasLength("") = false
* StringUtils.hasLength(" ") = true
* StringUtils.hasLength("Hello") = true
*
* @param str the CharSequence to check (may be null
)
* @return true
if the CharSequence is not null and has length
* @see #hasText(String)
*/
public static boolean hasLength(CharSequence str) {
return (str != null && str.length() > 0);
}
/**
* Count the occurrences of the substring in string s.
* @param str string to search in. Return 0 if this is null.
* @param sub string to search for. Return 0 if this is null.
*/
public static int countOccurrencesOf(String str, String sub) {
if (str == null || sub == null || str.length() == 0 || sub.length() == 0) {
return 0;
}
int count = 0;
int pos = 0;
int idx;
while ((idx = str.indexOf(sub, pos)) != -1) {
++count;
pos = idx + sub.length();
}
return count;
}
public static String composeMessage(String source, String... replacement) {
if (replacement.length == 0) {
return source;
}
for (int i = 0; i < replacement.length; i++) {
int start = source.indexOf("{");
if (start < 0) {
return source;
}
int end = source.indexOf("}") + 1;
String pattern = source.substring(start, end);
if(replacement[i]==null){
source = source.replace(pattern, "");
}else{
source = source.replace(pattern, replacement[i]);
}
}
return source;
}
}