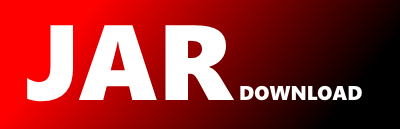
cn.tom.mvc.ext.BeanFactory Maven / Gradle / Ivy
package cn.tom.mvc.ext;
import java.util.concurrent.ConcurrentHashMap;
import cn.tom.mvc.core.CocookException;
/**
* 工厂
*/
public class BeanFactory {
public static final ConcurrentHashMap singletons = new ConcurrentHashMap();
public static T getInstance(Class _class){
String key = _class.getName();
if(singletons.containsKey(key)){
return _class.cast(singletons.get(key)) ;
}
try{
T tt = _class.newInstance();
singletons.put(key, tt);
return tt;
} catch (Exception e) {
throw new CocookException(e);
}
}
public static Object getInstance(String _class) {
if(singletons.containsKey(_class)){
return singletons.get(_class) ;
}
try{
Class> clazz = loadClass(_class);
Object tt = clazz.newInstance();
singletons.put(_class, tt);
return tt;
} catch (Exception e) {
throw new CocookException(e);
}
}
public static T newInstance(Class clazz){
try {
return clazz.newInstance();
} catch (Exception e) {
throw new CocookException(e);
}
}
public static Object newInstance(String _class){
try {
Class> clazz = loadClass(_class);
return clazz.newInstance();
} catch (Exception e) {
throw new CocookException(e);
}
}
public static Class> loadClass(String _clazz) throws ClassNotFoundException{
Class> clazz = BeanFactory.class.getClassLoader().loadClass(_clazz);
if(clazz == null){
clazz = Thread.currentThread().getContextClassLoader().loadClass(_clazz);
}
return clazz;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy