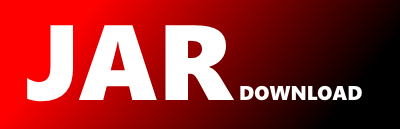
cn.tom.mvc.ext.Scan Maven / Gradle / Ivy
package cn.tom.mvc.ext;
import java.io.File;
import java.io.FileFilter;
import java.io.IOException;
import java.net.JarURLConnection;
import java.net.URL;
import java.net.URLDecoder;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import java.util.jar.JarEntry;
import java.util.jar.JarFile;
import cn.tom.mvc.config.Constants;
import cn.tom.mvc.core.CocookException;
public class Scan {
/**
* @param packName
* 要扫描的包
*/
public static Set> scanpackage(String packName) {
Set> clazzs = new LinkedHashSet>();
String packageDirName = packName.replace('.', '/');
Enumeration dirs;
try {
dirs = Thread.currentThread().getContextClassLoader().getResources(packageDirName);
while (dirs.hasMoreElements()) {
URL url = dirs.nextElement();
String protocol = url.getProtocol();
if ("file".equals(protocol)) {// 扫描file包中的类
String filePath = URLDecoder.decode(url.getFile(), Constants.getEncoding());
getFileClass(packName, filePath, clazzs);
} else if ("jar".equals(protocol)) {// 扫描jar包中的类
JarFile jarFile = ((JarURLConnection) url.openConnection()).getJarFile();
getJarClass(jarFile, packageDirName, clazzs);
}
}
} catch (Exception e) {
throw new CocookException(e);
}
return clazzs;
}
/**
* 获取文件中的class
*/
public static void getFileClass(String packName, String filePath, Set> clazzs) {
File dir = new File(filePath);
if (!dir.exists() || !dir.isDirectory()) {
throw new IllegalArgumentException("包目录不存在!");
}
File[] dirFiles = dir.listFiles(new FileFilter() {
public boolean accept(File file) {
return (file.isDirectory() || file.getName().endsWith(".class"));
}
});
for (File file : dirFiles) {
if (file.isDirectory()) {
getFileClass(packName + "." + file.getName(), file.getAbsolutePath(), clazzs);
} else {
String className = file.getName().substring(0, file.getName().length() - 6);
try {
Class> clazz = Thread.currentThread().getContextClassLoader().loadClass(packName + "." + className);
clazzs.add(clazz);
} catch (ClassNotFoundException e) {
throw new CocookException(e);
}
}
}
}
/**
* 获取jar中的class
*
* @throws IOException
*/
public static void getJarClass(JarFile jarFile, String filepath, Set> clazzs) throws IOException {
List jarEntryList = new ArrayList();
Enumeration enumes = jarFile.entries();
while (enumes.hasMoreElements()) {
JarEntry entry = (JarEntry) enumes.nextElement();
// 过滤出满足我们需求的东西
if (entry.getName().startsWith(filepath) && entry.getName().endsWith(".class")) {
jarEntryList.add(entry);
}
}
for (JarEntry entry : jarEntryList) {
String className = entry.getName().replace('/', '.');
className = className.substring(0, className.length() - 6);
//System.out.println("jarentry::"+className);
try {
clazzs.add(Thread.currentThread().getContextClassLoader().loadClass(className));
} catch (ClassNotFoundException e) {
throw new CocookException(e);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy