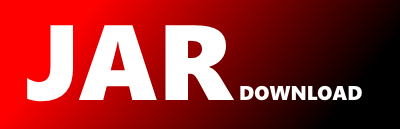
cn.tom.mvc.handler.CocookServlet Maven / Gradle / Ivy
package cn.tom.mvc.handler;
import java.io.IOException;
import java.util.Properties;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.*;
import cn.tom.mvc.annotation.HttpMethod;
import cn.tom.mvc.config.Constants;
import cn.tom.mvc.core.CocookException;
import cn.tom.mvc.core.DefaultLogConfigure;
import cn.tom.mvc.core.Initializers;
import cn.tom.mvc.core.RequestContext;
import cn.tom.mvc.interceptor.ActionInvocation;
import cn.tom.mvc.view.ViewFactory;
import cn.tom.db.jdbc.simple.DBUtil;
import cn.tom.db.mongo.MongoDao;
import cn.tom.kit.io.PropertiesUtil;
import cn.tom.kit.io.Resource;
@WebServlet(name="CocookServlet", urlPatterns={"/"}, loadOnStartup=1 )
public final class CocookServlet extends HttpServlet {
private static final long serialVersionUID = 2L;
private static final String SystemConfigLocation = "SystemConfigLocation" ;
private void initSystemConfig() throws IOException{
String app = getInitParameter(SystemConfigLocation);
if(app == null) app = "app.properties";
Resource resource = new Resource(app);
String webRoot = this.getServletContext().getRealPath("/");
resource.setWebRoot(webRoot);
Properties props = PropertiesUtil.loadProperties(resource);
props.put("webRoot", resource.getPhysicalwebapp());
Constants.getSyscfg().putAll(PropertiesUtil.toMap(props));
System.out.println(Constants.getSyscfg());
}
private void initLog(){
new DefaultLogConfigure().config();
}
private void initDataSource() throws IOException{
if("NONE".equals(Constants.getDBType())) return;
Resource resource = new Resource(Constants.getDBConfigLocation());
resource.setWebRoot(Constants.getWebRoot());
Properties props = PropertiesUtil.loadProperties(resource);
if("SQL".equals(Constants.getDBType())){
DBUtil.init(props);
return;
}
MongoDao.init(props);
}
private void initHandler() throws IOException, ClassNotFoundException{
new Initializers().scan();
}
private void initView(){
ViewFactory.initView();
}
@Override
public void init() throws ServletException {
try {
initSystemConfig();
initLog();
initDataSource();
initHandler();
initView();
} catch (Exception e) {
throw new CocookException(e);
}
}
@Override
public void destroy() {
super.destroy();
DBUtil.closeDataSource();
System.out.println("servlet destroy........");
}
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException {
process(RequestContext.begin(getServletContext(), req, res), HttpMethod.GET);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException {
process(RequestContext.begin(getServletContext(), req, res), HttpMethod.POST);
}
@Override
protected void doOptions(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException {
process(RequestContext.begin(getServletContext(), req, res), HttpMethod.OPTIONS);
}
@Override
protected void doPut(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doPost(req, resp);
}
@Override
protected void doDelete(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
private void process(RequestContext requestContext, HttpMethod method) throws ServletException, IOException {
try{
matchHandler(requestContext, method);
}finally{
if(requestContext!=null){
requestContext.end();
requestContext = null;
}
}
}
private void matchHandler(RequestContext context, HttpMethod method) throws ServletException, IOException{
String uri = context.getRequest().getRequestURI();
String contextPath = context.getRequest().getContextPath();
String queryAction = uri.substring(contextPath.length());
RequestMapping mapping = new RequestMapping();
mapping.setMethod(method);
mapping.setPath(queryAction);
try{
execHandler(mapping, context, method);
}catch(IOException e){
throw e;
}catch(ServletException ee){
throw ee;
}
}
private void execHandler(RequestMapping mapping, RequestContext context, HttpMethod method) throws ServletException, IOException{
for(Handler handler: Handler.handlers.values()){
HandlerInfo handlerInfo = handler.match(mapping, context);
if(handlerInfo == null) {
continue;
}
if(mapping.getMethod()!=null && mapping.getMethod()!= method) {
context.error(HttpServletResponse.SC_FORBIDDEN, mapping.toString());
return;
}
Controller controller = handler.proxyApp();
ActionInvocation actionInvocation = new ActionInvocation(handlerInfo, mapping);
if(actionInvocation.getMethod() == null){
continue;
}
controller.enter(actionInvocation);
controller = null;
return;
}
String msg = mapping + " NOT FOUND ON " + this.getServletName();
context.error(HttpServletResponse.SC_NOT_FOUND, msg);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy